How to use a named routes in a BottomNavigationBar in flutter?
9,884
Solution 1
Use Navigator.pushNamed()
onTap: (index){
switch(index){
case 0:
Navigator.pushNamed(context, "/first");
break;
case 1:
Navigator.pushNamed(context, "/second");
break;
...etc
}
},
Navigator.pushedName() needs context to find the Ancestor Widgets which contains the Navigator routing, now your BottomNavigationBar is already in the Root Widget-MaterialApp, which is not the Ancestor of the BottomNavigationBar, they are in the same context.
Solution 2
Just use pushNamed method of Navigator Navigator.pushNamed(context, 'Name'); https://api.flutter.dev/flutter/widgets/Navigator/pushNamed.html
onTap: (index){
switch (index) {
case 0:
Navigator.pushNamed(context, '/first');
break;
case 2:
Navigator.pushNamed(context, '/second');
break;
},
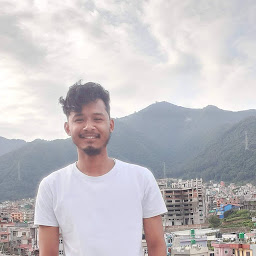
Author by
Aman Chaudhary
Updated on December 23, 2022Comments
-
Aman Chaudhary over 1 year
I am using
BottomNavigationBar
and when clicked on any icons in the navigation bar. I want it to go to next screen. That's why I am using named route here.main.dart
codeimport 'package:flutter/material.dart'; import 'Screens/profilePage1/profilePage1.dart'; void main() => runApp(MyStatefulWidget()); class MyStatefulWidget extends StatefulWidget { @override _MyStatefulWidgetState createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { int _selectedIndex = 0; void _onItemTapped(int index) { setState(() { _selectedIndex = index; }); } @override Widget build(BuildContext context) { return MaterialApp( routes: { // When navigating to the "/" route, build the FirstScreen widget. '/first': (context) => ProfilePage1(), // When navigating to the "/second" route, build the SecondScreen widget. '/second': (context) => ProfilePage1(), '/third': (context) => ProfilePage1(), '/fourth': (context) => ProfilePage1(), '/fifth': (context) => ProfilePage1(), }, home: Scaffold( body: Center( child: routes.elementAt(_selectedIndex), ), bottomNavigationBar: BottomNavigationBar( items: <BottomNavigationBarItem>[ BottomNavigationBarItem(icon: Icon(Icons.home), title: Text("")), BottomNavigationBarItem( icon: Icon(Icons.calendar_today), title: Text("")), BottomNavigationBarItem( icon: Icon( Icons.account_circle, size: 35, color: Color(0xFF334192), ), title: Text("")), BottomNavigationBarItem(icon: Icon(Icons.message), title: Text("")), BottomNavigationBarItem( icon: Icon(Icons.table_chart), title: Text("")), ], currentIndex: _selectedIndex, selectedItemColor: Color(0xFF334192), unselectedItemColor: Colors.grey, onTap: (index){ }, ), ), ); } }
Here I have created 5 named routes. And I want it to pass to body when particular tab is clicked. How do I do that?
-
Aman Chaudhary almost 4 yearswhy is it giving this error? Can you help me with this? Navigator operation requested with a context that does not include a Navigator. The context used to push or pop routes from the Navigator must be that of a widget that is a descendant of a Navigator widget.
-
sleepingkit almost 4 years@AmanChaudhary Extract your Scaffold Widget to a Stateless/Stateful Widget may solve the error
-
Aman Chaudhary almost 4 yearsthis did solve the problem but what was the reason behind this?
-
Bancha Rajainthong almost 4 years@Aman Chaudhary This because your 'MyStatefulWidget' class doesn't have a MaterialApp as a parent. You can take a look for more explanation from Rémi Rousselet here. stackoverflow.com/a/51292613/7610338
-
Alexandre Jean about 3 yearsIs also good practice with a switch to have a default: that goes to home screen/page or an error screen/page. stackoverflow.com/questions/4649423/…