How to use function srand() with time.h?
Solution 1
You need to call srand()
once, to randomize the seed, and then call rand()
in your loop:
#include <stdlib.h>
#include <time.h>
#define size 10
srand(time(NULL)); // randomize seed
for(i=0;i<size;i++)
Arr[i] = rand()%size;
Solution 2
Try to call randomize() before rand() to initialize random generator.
(look at: srand() — why call it only once?)
Solution 3
If you chose to srand
, it is a good idea to then call rand()
at least once before you use it, because it is a kind of horrible primitive psuedo-random generator. See Stack Overflow question Why does rand() % 7 always return 0?.
srand(time(NULL));
rand();
//Now use rand()
If available, either random
or arc4rand
would be better.
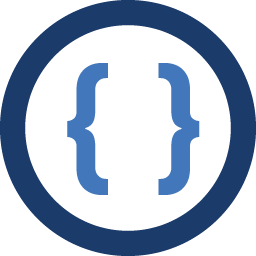
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
My program contains code that should generate a random positive integer number every time I execute it. It generates random numbers but only once. After that, when I execute same code, it gives me same values, and it is making my code useless.
I started with the rand function, and then I used the srand() function with the time.h header file, but still it is not working properly.
#define size 10 for(i=0;i<size;i++) Arr[i] = rand()%size;
First call (random):
6 0 2 0 6 7 5 5 8 6
Second call (random but same as previous):
6 0 2 0 6 7 5 5 8 6
Later I visited Stack Overflow questions and I read about the srand() function, and I used it as:
#include<time.h> for(i=0;i<size;i++) Arr[i] = srand(time(NULL));
First call:
-10327 -10327 -10327 -10327 -10327 -10327 -10327 -10327 -10327 -10327
Second call:
-10326 -10326 -10326 -10326 -10326 -10326 -10326 -10326 -10326 -10326
It is giving me different (but not random values). I've defined Arr[i] as unsigned int, and still I am getting negative values.
-
Admin almost 11 yearsPlease write me the code, it would be really helpful if you put it
-
Paul R almost 11 years
randomize
is not a standard function - perhaps you meansrand
? -
Ze.. almost 11 yearsPaul R, yes, i mean srand() from the "stdlib.h". Thank you for adding comment. My mistake.
-
Ze.. almost 11 yearscplusplus.com/reference/cstdlib/srand has an example where srand is called more than once.
-
Daniel Fischer almost 11 yearsThat example is to illustrate that not calling
srand()
has the same effect as callingsrand(1)
. It should be made clearer, though. -
Ze.. almost 11 years@Ðeepak, may this information will help you: stackoverflow.com/questions/7343833/srand-why-call-only-once
-
Ze.. almost 11 years@Daniel Fischer, thank you for the hint.
-
R.M.VIVEK Arni over 9 yearsthis method encryption and decryption progamming used subpart
-
Jiminion about 7 yearssrand() takes an unsigned int as an input, so time(NULL) should be recast.