How to use mpi on Mac OS X
Solution 1
If I were you, I'd do following:
Installation of Open MPI is fairly straightforward
https://www.open-mpi.org/software/ompi/v2.0/
> tar xf openmpi-2.0.2.tar
> cd openmpi-2.0.2/
> ./configure --prefix=$HOME/opt/usr/local
> make all
> make install
> $HOME/opt/usr/local/bin/mpirun --version
mpirun (Open MPI) 2.0.2
Report bugs to http://www.open-mpi.org/community/help/
You can use super simple hello world for testing purposes
#include <mpi.h>
#include <stdio.h>
int main(int argc, char** argv) {
MPI_Init(NULL, NULL);
int rank;
int world;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &world);
printf("Hello: rank %d, world: %d\n",rank, world);
MPI_Finalize();
}
After everything is in place, simply compile and run the code
$HOME/opt/usr/local/bin/mpicc -o hello ./hello.c
$HOME/opt/usr/local/bin/mpirun -np 2 ./hello
Hello: rank 0, world: 2
Hello: rank 1, world: 2
Works perfectly fine on macOS
Update
In case you are looking for MPI
related environment on macOS, I suggest checking out concept of modules
.
- building MPICH/OpenMPI - https://www.owsiak.org/building-opencoarrays-on-macos-everything-from-the-sources-gcc-9-2-0/
- modules - https://www.owsiak.org/modules-as-a-convenient-way-of-choosing-build-chain-on-macos/
Solution 2
Building on the answer provided by @Oo.oO.
Install OpenMPI
.
It can be installed using brew
.
brew install openmpi
Solution 3
On some versions of Mac OS X / MacOS Sierra, the default temporary directory location is sufficiently long that it is easy for an application to create file names for temporary files which exceed the maximum allowed file name length, Which can cause this error.
so you should set the temporary directory using export TMPDIR=/tmp
more details here https://www.open-mpi.org/faq/?category=osx
Solution 4
If you have anaconda installed, this is the fastest option for me:
conda install mpi4py openmpi
Solution 5
I have noticed that during execution pmix offers an unnecesary exception of the form:
PMIX ERROR: ERROR in file gds_ds12_lock_pthread.c at line 206
To prevent this, before the execution of main file always type this on the terminal prompt :
export PMIX_MCA_gds=hash
This helps subside the pmix noice and has to be repeated every session.
Note: The maximum number of slots that pmi allows is by default 2 in macs. A "slot" is the Open MPI term for an allocatable unit where we can launch a process. This determines how many time we can run an instruction in a code. To extend the number of slots carry out the following steps:
1.Create a hostfile with anyname
2.within the write:
localhost slots = <#>
where #=no. of slots needed.
while compiling write the following on the terminal prompt:
mpicc -hostfile -<filename>
While running wite the following on the terminal prompt:
mpirun -hostfile -np <#>
Although be weary of the last note,it isnt often needed and the file extention of the hostfile may become a problem with certain architectures , such as my own x86.
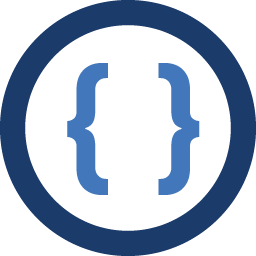
Admin
Updated on October 14, 2020Comments
-
Admin over 3 years
I have been searching for a way to use mpi on my mac but everything is very advanced.
I have successfully installed open-mpi using
brew install open-mpi
I have .c files ready for compiling and running. When I type:
mpicc -o <file> <file.c>
followed by
mpirun <file>
I get
[xxxxx-MacBook-Pro.local:13623] [[44919,0],0] ORTE_ERROR_LOG: Bad parameter in file orted/pmix/pmix_server.c at line 262
[xxxxx-MacBook-Pro.local:13623] [[44919,0],0] ORTE_ERROR_LOG: Bad parameter in file ess_hnp_module.c at line 666It looks like orte_init failed for some reason; your parallel process is likely to abort. There are many reasons that a parallel process can fail during orte_init; some of which are due to configuration or environment problems. This failure appears to be an internal failure; here's some additional information (which may only be relevant to an Open MPI developer):
pmix server init failed
--> Returned value Bad parameter (-5) instead of ORTE_SUCCESSI also get the same message when I enter:
mpirun -np 2 <file>
What am I doing wrong?