how to use powershell to copy file and retain original timestamp
Solution 1
Here's a powershell function that'll do what you're asking... It does absolutely no sanity checking, so caveat emptor...
function Copy-FileWithTimestamp {
[cmdletbinding()]
param(
[Parameter(Mandatory=$true,Position=0)][string]$Path,
[Parameter(Mandatory=$true,Position=1)][string]$Destination
)
$origLastWriteTime = ( Get-ChildItem $Path ).LastWriteTime
Copy-Item -Path $Path -Destination $Destination
(Get-ChildItem $Destination).LastWriteTime = $origLastWriteTime
}
Once you've run loaded that, you can do something like:
Copy-FileWithTimestamp foo bar
(you can also name it something shorter, but with tab completion, not that big of a deal...)
Solution 2
Here is how you can copy over the time stamps, attributes, and permissions.
$srcpath = 'C:\somepath'
$dstpath = 'C:\anotherpath'
$files = gci $srcpath
foreach ($srcfile in $files) {
# Build destination file path
$dstfile = [io.FileInfo]($dstpath, '\', $srcfile.name -join '')
# Copy the file
cp $srcfile.FullName $dstfile.FullName
# Make sure file was copied and exists before copying over properties/attributes
if ($dstfile.Exists) {
$dstfile.CreationTime = $srcfile.CreationTime
$dstfile.LastAccessTime = $srcfile.LastAccessTime
$dstfile.LastWriteTime = $srcfile.LastWriteTime
$dstfile.Attributes = $srcfile.Attributes
$dstfile.SetAccessControl($srcfile.GetAccessControl())
}
}
Solution 3
If you are okay with a two-step solution; then
- First copy files over from source to dest
- loop over each file; and each attribute for each file
- copy attributes from source to destination
Try this technique to copy file attributes from one file to another. (I have illustrated this with LastWriteTime; I am sure you can extend it for other attributes).
#Created two dummy files
PS> echo hi > foo
PS> echo there > bar
# Get attributes for first file
PS> $timestamp = gci "foo"
PS> $timestamp.LastWriteTime
06 February 2014 09:25:47
# Get attributes for second file
PS> $t2 = gci "bar"
PS> $t2.LastWriteTime
06 February 2014 09:25:53
# Simply overwrite
PS> $t2.LastWriteTime = $timestamp.LastWriteTime
# Ta-Da!
PS> $t2.LastWriteTime
06 February 2014 09:25:47
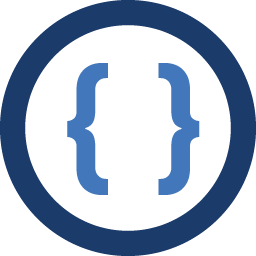
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
I would like to copy some files or a folder of files from one file server to another. However, I want to keep the original timestamp and file attributes so that the newly copied files will have the same timestamp of the original files. Thanks in advance on any answer.
-
Guy Thomas over 10 yearsNeat. It worked when I tested Copy-FileWithTimestamp.
-
Hunter Eidson over 10 yearsI haven't tested it, but I would expect it to fail spectacularly if you tried to use a wildcard in the copy the way I wrote that function...
-
Guy Thomas over 10 yearsI merely wanted to help the community by reporting that I tried your code and it worked. I did not mean to imply that I was giving an endorsement for use in a production network.
-
Hunter Eidson over 10 yearsNo problem... my comment was more of an afterthought when I was driving into the office today... :)
-
jpaugh over 7 yearsUsing
Get-Item
would probably be safer, especially if$Destination
is a directory. -
stackprotector over 2 yearsNice reminder to take into account all three timestamps and not just
LastWriteTime
.