How to use Random Randint for List ?
Solution 1
You wouldn't use random.randint()
at all. You'd use random.choice()
instead:
import random
questions = [question1, question2, question3]
random_question = random.choice(questions)
The function picks one element at random from a sequence.
If you need to produce questions at random without repetition, you want to do something different; you would use random.shuffle()
to randomize the whole list of questions, then just pick one from that list (perhaps removing it from the list) every time you need a new question. That produces a random sequence of questions.
import random
questions = [question1, question2, question3]
random.shuffle(questions)
for question in questions:
# questions are iterated over in random order
or
questions = [question1, question2, question3]
random.shuffle(questions)
while questions:
next_question = questions.pop()
Solution 2
Don't use randint
, use random.choice
. This function will select a random item from a list.
import random
l = [1,2,3]
>>> random.choice(l)
2
>>> random.choice(l)
1
>>> random.choice(l)
1
>>> random.choice(l)
3
Solution 3
If you need to use randint
because of an assignment, you can look at how choice
works. Slightly simplified, it's this:
def choice(seq):
i = randrange(len(seq))
return seq[i]
And randint(a, b)
is just "an alias for randrange(a, b+1)
".
So, you know how to use choice
from Martijn Pieter's answer, you know what choice
does, you should be able to figure out from there how to use randint
from there.
Solution 4
If "you want to pick with replacement (that is, each time there's a 1/3 chance of each)":
#!/usr/bin/env python
import random
questions = ["question1", "question2", "question3"]
while True: # infinite loop, press Ctrl + C to break
print(random.choice(questions))
"without replacement (that is, each one only shows up once, so after 3 choices there's nothing left)":
#!/usr/bin/env python
import random
questions = ["question1", "question2", "question3"]
random.shuffle(questions)
while questions: # only `len(questions)` iterations
print(questions.pop())
"or some hybrid (e.g., pick all 3 in random order, then repeat all 3 in random order again, etc.":
#!/usr/bin/env python
import random
questions = ["question1", "question2", "question3"]
while True: # infinite loop, press Ctrl + C to break
random.shuffle(questions)
for q in questions: # only `len(questions)` iterations
print(q)
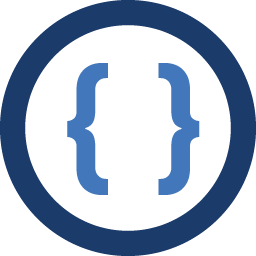
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
I wrote 3 Questions and I want to use Random Randint to pick and display one by one randomly. I don't know how to use Random Randint to use that in my Code.