How to write localStorage data to a text file in Chrome
Solution 1
If you don't want to use a server-side solution (does not have to be PHP of course), the easiest way is to use a data URI:
data:text/plain,Your text here
This will show the text file in the browser, and let the user save it where they want. I don't think it's possible to show a "Save as"-dialog for those kind of URI:s.
Note: this requires IE8 at least. But I'm guessing that's your requirement anyway, since you are using localStorage.
Solution 2
I found this console.save(data, [filename]) method you can add to the console that does the trick pretty easily. Note that when the file is downloaded it just goes directly into the default downloads folder. To add it simply run:
(function(console){
console.save = function(data, filename){
if(!data) {
console.error('Console.save: No data')
return;
}
if(!filename) filename = 'console.json'
if(typeof data === "object"){
data = JSON.stringify(data, undefined, 4)
}
var blob = new Blob([data], {type: 'text/json'}),
e = document.createEvent('MouseEvents'),
a = document.createElement('a')
a.download = filename
a.href = window.URL.createObjectURL(blob)
a.dataset.downloadurl = ['text/json', a.download, a.href].join(':')
e.initMouseEvent('click', true, false, window, 0, 0, 0, 0, 0, false, false, false, false, 0, null)
a.dispatchEvent(e)
}
})(console)
Then pass in the data and filename like so, console.save(data, [filename]) and the file will be created and downloaded.
Source:
https://plus.google.com/+AddyOsmani/posts/jBS8CiNTESM
http://bgrins.github.io/devtools-snippets/#console-save
Solution 3
Since the question is tagged with google-chrome-extension I want to provide a simpler solution for extension developers.
First, add "downloads" to permissions in manifest.json
"permissions": [
"downloads"
]
Then use the downloads-API with the data:text/plain
trick as provided by @emil-stenström.
var myString = localStorage.YOUR_VALUE;
chrome.downloads.download({
url: "data:text/plain," + myString,
filename: "data.txt",
conflictAction: "uniquify", // or "overwrite" / "prompt"
saveAs: false, // true gives save-as dialogue
}, function(downloadId) {
console.log("Downloaded item with ID", downloadId);
});
To adapt for JSON, just prepare your object or array with JSON.stringify(localStorage.YOUR_DATA)
, then use data:text/json
and change the file ending to .json
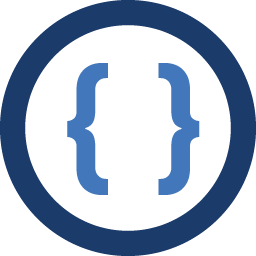
Admin
Updated on July 12, 2022Comments
-
Admin almost 2 years
I want to write a localStorage item to a text file and want to invoke user to store the file in a specified location. Please help me with extending the code
var data = JSON.parse(localStorage.getItem(pid)); var Text2Write = ""; for(var pr in ele) { var dat = pr + ":" + ele[pr] + "\n"; Text2Write += dat; } // Here I want to store this Text2Write to text file and ask user to save where he wants.
Please help me extending this code.
-
Emil Stenström over 12 yearswindow.location = "data:text/plain,Your text here";
-
Admin over 12 years@Emilstenstorm Like this? $('#but').click(function(){ window.location = "data:text/plain, My Data" });