How would I create a hex dump utility in C++?
17,076
I don't normally do this for your kind of question.... But it doesn't take much to knock something like this up, and maybe you can learn from it. Here's a no-frills program that just reads from standard input and outputs in roughly the same format as you showed. Try it out here.
Code
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
unsigned long address = 0;
cout << hex << setfill('0');
while( cin.good() )
{
int nread;
char buf[16];
for( nread = 0; nread < 16 && cin.get(buf[nread]); nread++ );
if( nread == 0 ) break;
// Show the address
cout << setw(8) << address;
// Show the hex codes
for( int i = 0; i < 16; i++ )
{
if( i % 8 == 0 ) cout << ' ';
if( i < nread )
cout << ' ' << setw(2) << (unsigned int)(unsigned char)buf[i];
else
cout << " ";
}
// Show printable characters
cout << " ";
for( int i = 0; i < nread; i++)
{
if( buf[i] < 32 ) cout << '.';
else cout << buf[i];
}
cout << "\n";
address += 16;
}
return 0;
}
Input
Hello there, this is a test binary file.
What do you think?
.
Output
00000000 48 65 6c 6c 6f 20 74 68 65 72 65 2c 20 74 68 69 Hello there, thi
00000010 73 20 69 73 20 61 20 74 65 73 74 20 62 69 6e 61 s is a test bina
00000020 72 79 20 66 69 6c 65 2e 0a 57 68 61 74 20 64 6f ry file..What do
00000030 20 79 6f 75 20 74 68 69 6e 6b 3f 0a 0a 2e you think?...
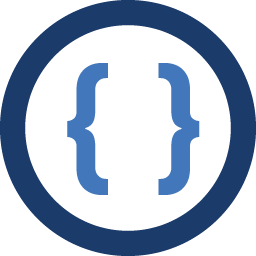
Author by
Admin
Updated on August 10, 2022Comments
-
Admin over 1 year
Basically, I need to write a hex dump utility using C++. It'll look something like this
(Part of a Word document's hex dump using Visual Studio)
I want to prompt the user for a file name, and then display the hexadecimal values as well as the translated ASCII characters. I'm still new at working with binary files, so if you could keep it simple, that would be much appreciated.
-
Admin almost 11 yearsAh ok, so you need to use a buffer. Thank you for the help.
-
Wolf almost 7 yearsWhat is
c
for? -- just a mascot? ;-) -
Wolf almost 7 yearsThis code depends on whether the default char type is being signed or not.