HTML anchor link with no scroll or jump
Solution 1
I'm probably missing something, but why not just give them different IDs?
<a href="#button1" id="button-1">button 1</a>
<a href="#button2" id="button-2">button 2</a>
<a href="#" id="reset">Home</a>
Or whatever convention you'd prefer.
Solution 2
The whole point of an anchor link is to scroll a page to a particular point. So if you don't want that to happen, you need to attach an onclick
handler and return false. Even just adding it as an attribute should work:
<a href="#button1" id="button1" onclick="return false">button 1</a>
A side of effect of the above is that the URL itself won't change, since returning false will cancel the event. So since you want the URL to actually change, you can set the window.location.hash
variable to the value that you want (that is the only property of the URL that you can change without the browser forcing a reload). You can probably attach an event handler and call something like window.location.hash = this.id
though I'm not sure how mootools handles events.
(Also you need all of the IDs to be unique)
Solution 3
You can use the code below to avoid scrolling:
<a href="javascript:void(0);">linktxt</a>
Solution 4
Also, preventDefault
$(your-selector).click(function (event) {
event.preventDefault();
//rest of your code here
}
Solution 5
I found the solution. Here I save an old location from calling href and restore it after scrolling
<script language="JavaScript" type="text/javascript">
<!--
function keepLocation(oldOffset) {
if (window.pageYOffset!= null){
st=oldOffset;
}
if (document.body.scrollWidth!= null){
st=oldOffset;
}
setTimeout('window.scrollTo(0,st)',10);
}
//-->
</script>
and in body of page
<a href="#tab1" onclick="keepLocation(window.pageYOffset);" >Item</a>
Thanks to sitepoint
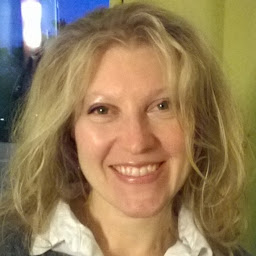
Elisa74
I’m an Mobile Engineer in San Francisco who loves Objective-C and exploring new user interfaces.
Updated on April 14, 2021Comments
-
Elisa74 about 3 years
I have various links which all have unique id's that are "pseudo-anchors." I want them to affect the url hash value and the click magic is all handled by some mootools code. However, when I click on the links they scroll to themselves (or to the top in one case). I don't want to scroll anywhere, but also need my javascript to execute and to have the hash value in the url update.
Simulated sample code:
<a href="#button1" id="button1">button 1</a> <a href="#button2" id="button2">button 2</a> <a href="#" id="reset">Home</a>
So if you were to click on the "button 1" link, the url could be http://example.com/foo.php#button1
Does anyone have any ideas for this? Simply having some javascript return void kills the scrolling but also kills my javascript (though I could probably work around that with an onclick) but more importantly, prevents the hash value in the url to change.