HTML Canvas image to Base64 problem
Your document.write()
call occurs immediately upon page load, before your img
is loaded and drawn and before your onclick
handler can ever run. You need to wait to generate the data URI until after everything has finished happening to the canvas.
Ignoring the onclick
, here's something that writes out the data url after the image has loaded and been drawn to the canvas in a rotated fashion:
<!DOCTYPE html>
<html><head>
<title>Rotated Image Data URL</title>
<style type="text/css" media="screen">
canvas, textarea { display:block }
</style>
</head><body>
<canvas id="canvas"></canvas>
<textarea id="data" rows="20" cols="80"></textarea>
<img id="echo">
<script type="text/javascript">
var can = document.getElementById('canvas');
var ctx = can.getContext('2d');
var img = new Image();
img.onload = function(){
can.width = img.width;
can.height = img.height;
ctx.translate(img.width-1, img.height-1);
ctx.rotate(Math.PI);
ctx.drawImage(img, 0, 0, img.width, img.height);
var url = document.getElementById('data').value = can.toDataURL();
document.getElementById('echo').src = url;
}
img.src = 'gkhead.jpg';
</script>
</body></html>
You can see this demo in action here: http://phrogz.net/tmp/upside-down-image-url.html
Note that the image you load must be on the same domain as your script, or else you will not be allowed to create the data URL.
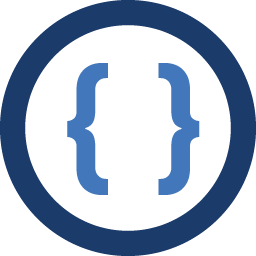
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm having a problem with retrieving the base64 version of an image displayed on a canvas. I've looked at the other questions but none of the solutions including canvas2image seem to work.
Here's my code:
<!DOCTYPE> <html> <head> <style>#canvas {cursor: pointer;}</style> </head> <body> <canvas id="canvas"></canvas> <script type="text/javascript"> var can = document.getElementById('canvas'); var ctx = can.getContext('2d'); var img = new Image(); img.onload = function(){ can.width = img.width; can.height = img.height; ctx.drawImage(img, 0, 0, img.width, img.height); } img.src = 'http://sstatic.net/stackoverflow/img/newsletter-ad.png'; can.onclick = function() { ctx.translate(img.width-1, img.height-1); ctx.rotate(Math.PI); ctx.drawImage(img, 0, 0, img.width, img.height); }; document.write(can.toDataURL()); //isn't writing the correct base64 image </script> </body> </html>
Here's the test link: http://jsfiddle.net/mrchief/hgTdM/1/ thanks, Mrchief
What I need is the correct base64 output of the image in the canvas.
The problem is that it won't output the correct base64 version of the image.. But as you can see, the image inside the canvas is displayed without any problems.
I've tested it on chrome & firefox
Thanks very much..
-
Admin over 12 yearsThanks phrogz. The problem was that the image was on a different domain. I had to use php to solve that.