Html.Partial not rendering partial view
Solution 1
It is actually razor syntax to tell that we are starting to write c# code, if your don't put @ it will be considered as plain text so you need to put @ sign before writing c# code in the view and in html helper method you don't need to put semicolon in front of then it is razor syntax to write helpers this way.
For Example:
@Html.LabelFor(x=>m.SomeProperty) // here @ is telling that we are writing c# statement
When you write:
@if (SiteSession.SubPageHelper.DisplayType == DisplayType.List)
{
Html.Partial("_SubLandingPage_List"); // this is wrong syntax
}
else
{
Html.Partial("_SubLandingPage_Grid");
}
the right way is to tell that this is a razor html helper and c# statement:
@if (SiteSession.SubPageHelper.DisplayType == DisplayType.List)
{
@Html.Partial("_SubLandingPage_List")
}
else
{
@Html.Partial("_SubLandingPage_Grid")
}
you can see more on razor syntax HERE
Few more links which will help you understanding razor:
http://weblogs.asp.net/scottgu/asp-net-mvc-3-razor-s-and-lt-text-gt-syntax
http://weblogs.asp.net/scottgu/introducing-razor
UPDATE:
An alternative can be to use RenderPartial which will work in the if statement without putting @ sign:
@if (SiteSession.SubPageHelper.DisplayType == DisplayType.List)
{
Html.RenderPartial("_SubLandingPage_List");
}
else
{
Html.RenderPartial("_SubLandingPage_Grid");
}
For understanding Difference between Html.Partial
and Html.RenderPartial
, visist these links:
Html.Partial vs Html.RenderPartial & Html.Action vs Html.RenderAction
Solution 2
Well simply the "@" symbol instructs Razor that it have to render something. The @ always expect something to print from the supplied statement. If you don't want to use this syntax you can still render the content with minor changes in your code in loop .. look at this.
@if (SiteSession.SubPageHelper.DisplayType == DisplayType.List)
{
Html.RenderPartial("_SubLandingPage_List"); // this is wrong syntax
}
else
{
Html.RenderPartial("_SubLandingPage_Grid");
}
Html.RenderPartial
will directly write it to stream and doesn't requires @.
Even if you try to write something like following
@Html.RenderPartial("_SubLandingPage_Grid")
This will give you an error as @ expect something to return and Html.RenderPartial
returns void
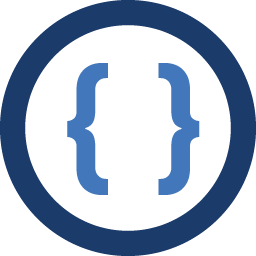
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
I have the following code in a view:
@if (SiteSession.SubPageHelper.DisplayType == DisplayType.List) { Html.Partial("_SubLandingPage_List"); } else { Html.Partial("_SubLandingPage_Grid"); }
and within the partials I just have a foreach loop like this:
@foreach (Product product in SiteSession.SubPageHelper.PagedProducts) { some html code here }
Where
PagedProducts
is got from doing a.Take()
on a cached list of productsNow the above code doesn't display my paged products but if I change the partial to include the at symbol remove the semi-colon:
@Html.Partial("_SubLandingPage_Grid")
It will display the products properly. Can anyone tell me what the difference between the two version are as it took me ages to figure out why the products weren't displaying