If statement with && and multiple OR conditions in PHP
&& takes precedence over ||, thus you would have to use parentheses to get the expected result:
if (isset($_POST['cookieGiftBox']) && (!isset($POST['sugar']) || ...)
Actually, to get check if nothing is selected, you would do something like this:
if checkbox is checked and not (sugar is checked or chocolatechip is checked)
or the equivalent:
if checkbox is checked and sugar is not entered and chocolatechip is not entered...
.
If you want to know more, search for information about Boolean algebra.
Update: In your example and in the correct syntax the sentence I took as an example would like this (for the first sentence, note the not (!) and the parentheses around the fields, not the checkbox):
if (isset($_POST['cookieGiftBox']) &&
!(
isset($_POST['sugar']) ||
isset($_POST['chocolatechip'])
)
) { error ...}
Or the second sentence, which might be easier to understand (note the && instead of ||):
if (isset($_POST['cookieGiftBox']) &&
!isset($_POST['sugar']) &&
!isset($_POST['chocolatechip'])
) { error...}
Using ands makes sure it is only true (and thus showing the error) if none of the sugar, chocolatechips, etc. fields are set when the giftbox checkbox is checked.
So if the checkbox is checked, and no fields are set it looks like this:
(true && !false && !false)
which is equivalent to (true && true && true)
which is true
, so it shows the error.
If the checkbox is checked and sugar is entered it would look like this:
(true && !true && !false)
, equ. to (true && false && true)
, equ. to false
and thus no error is shown.
If both sugar and chocolatechip are entered also no error will be shown.
Related videos on Youtube
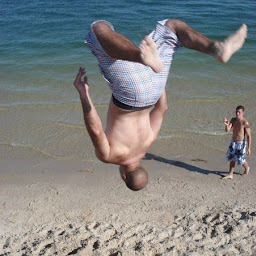
Dustin Galbreath
Updated on June 04, 2022Comments
-
Dustin Galbreath almost 2 years
I'm having trouble trying to make the statement below work the way I want it to.
I am trying to display an error message for an order form if at least one text field is not filled out. Below is a snippet of my PHP code. The 'cookieGiftBox' is the name of a checkbox that users can select and if it is selected, they must enter an amount of cookies under their desired flavors in the text fields provided. How can I display an error message to display when the checkbox IS selected but NO text fields have been filled out?
<?php if (isset($_POST['cookieGiftBox']) && (!isset($_POST['sugar']) || ($_POST['chocolateChip']) || ($_POST['chocolateChipPecan']) || ($_POST['whiteChocolateRaspberry']) || ($_POST['peanutChocolateChip']) || ($_POST['peanutButter']) || ($_POST['tripleChocolateChip']) || ($_POST['whiteChocolateChip']) || ($_POST['oatmealRaisin']) || ($_POST['cinnamonSpice']) || ($_POST['candyChocolateChip']) || ($_POST['butterscotchToffee']) || ($_POST['snickerdoodle']))) { $error.="<br />Please enter an Amount for Cookie Flavors"; } ?>
-
Dustin Galbreath over 8 yearsI appreciate the response, however I have not been able to get it working. Based off your example, I believe I have all the parentheses in the correct places.
if (isset($_POST['cookieGiftBox']) && (!$_POST['sugar']) || (!$_POST['chocolateChip'])) {
here is a shortened version of what I have. This code displays an error if checkbox is checked and no text entered in boxes. However, both 'sugar' AND 'chocolateChip' text fields need to be filled to clear error message. I would like to have it where only one field needs to be filled. So one OR the other...How can I do this? Thank you -
Ayonix over 8 yearsYour condition is true if either chocolatechip is not set or the checkbox is checked and sugar isn't set (translated it one to one). I updated the answer with two ways of having your problem solved.