ifstream is failing to open file
Solution 1
when using the "\" to define a path use two instead of one C:\ \scores.txt
Solution 2
try this:
#include<iostream>
#include<fstream>
#include<string>
using namespace std;
void getHighScores(int scores[], string names[]);
int main()
{
string filename = "scores.txt"; // could come from command line.
ifstream fin(filename.c_str());
if (!fin.is_open())
{
cout << "Could not open file: " << filename << endl;
return 1;
}
int scores[32];
string names[32];
int iter = 0;
while (fin >> names[iter] >> scores[iter])
{
if (++iter >= 32 )
{
break;
}
cout << iter << endl;
}
if (iter >= 2)
{
cout << scores[2] << endl;
}
fin.close();
return 0;
}
void getHighScores(int scores[], string names[])
{
}
Solution 3
Had me stumped for a bit. Your C++ code is reading scores and names in the opposite order from your input text. The first line of text in the input file is Ronaldo
, but your first operator>>
is to score[0]
(an int
). This causes the failbit
to be set, and so fail()
returns true
. It also explains why you end up getting garbage for the destination array elements.
Reverse the order of the scores/names in either the scores.txt
file or your C++ parsing code (but not both!) and you should be good to go.
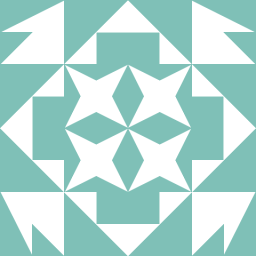
user3116382
Updated on November 25, 2022Comments
-
user3116382 about 1 month
Here is my code:
#include<iostream> #include<fstream> #include<string> using namespace std; void getHighScores(int scores[], string names[]); int main() { ifstream stream; stream.open("scores.txt"); int scores[32]; string names[32]; stream>>scores[0]; stream>>names[0]; if(stream.fail()) cout<<"It failed\n"<<strerror(errno)<<endl; for(int i=1;i<5;i++) { stream>>scores[i]; stream>>names[i]; cout<<i<<endl; } cout<<scores[2]<<endl; stream.close(); return 0; } void getHighScores(int scores[], string names[]) { }
It get garbage output for scores[2] because stream.open("scores.txt") fails to open a file. strerror(errno) gives me "No error".
I've checked to see if my file is really called "scores.txt.txt". It is not. I've also tried moving my file to "C:\scores.txt". I've tried using the full address. I've tried deleting it and re-creating it. I've tried other things too that I cannot remember. ![enter image description here][1]I've been trying for hours to fix this and I'm desperate. I'd be grateful if anyone could help me fix this.
void gethighscores is a function that I plan to use later.
The input file looks like this:
Ronaldo 10400 Didier 9800 Pele 12300 Kaka 8400 Cristiano 8000
The output of the program looks like this
It failed No error 1 2 3 4 -858993460 Press any key to continue . . .
I'm running this in Microsoft Visual Studio Express 2012 for Windows Desktop My operating system is Windows 7 ultimate 64 bit.
-
MooseBoys about 9 yearsAre you sure that scores.txt actually exists in your working directory? It's not necessarily the path of the executable. Also, trying to open a file directly from C:\ may fail for other reasons (e.g. permissions). Also, you should check for open failure (
stream.is_open()
) immediately after calling open (not only after trying to read). -
WhozCraig about 9 yearsIt would probably assist you considerably to actually check the validity of you stream extractions rather than simply blindly assuming they work. Also, a real sample of your input file as well as the real output you're receiving would improve this question substantially, along with the platform you're running on.
-
James Kanze about 9 years@WhozCraig The fact that he tried
"C:\scores.txt"
is a pretty good hint about the platform. -
Slava about 9 years@JamesKanze but was path really "C:\\scores.txt" that is the question
-
user3116382 about 9 years@Mooseboys when I change the name or directory the file to something that does not exist, I get "No such file or directory" from errno. I'm pretty sure because of that, that the working directory and the name of the file are not the problem.
-
James Kanze about 9 years@Slava That's a possibility. The fact that he doesn't check the results immediately is clearly wrong, though. Whether it is after the open, or after each input.
-
-
user3116382 about 9 yearsJust tried this. I just added the lane that declares the score variable
int score[5];
since you forgot it. I got the following with your code:Could not open file: score.txt Press any key to continue . . .
-
MooseBoys about 9 yearsDownvoted.
scores[0]
andnames[0]
do not need an initial value prior to writing them from the stream. Verified that removing the two lines does not solve the problem. -
Chad Befus about 9 yearsTry putting "score.txt" in the same directory as the executable you are running (at the base of the project).
-
Chad Befus about 9 yearsI confirmed that the above code loads the file (with the content you posted above) and does exactly as expected. The scores.txt file was placed in the same location as the executable.
-
James Kanze about 9 yearsNot
badbit
, butfailbit
.