Put A String In A ifstream Method
15,520
Solution 1
You should pass char*
to ifstream
constructor, use c_str()
function.
// includes !!!
#include <fstream>
#include <iostream>
#include <string>
using namespace std;
int main()
{
string filename;
cout << "Enter the name of the file: ";
cin >> filename;
ifstream file ( filename.c_str() ); // c_str !!!
}
Solution 2
The problem is that ifstream's constructor does not accept a string, but a c-style string:
explicit ifstream::ifstream ( const char * filename, ios_base::openmode mode = ios_base::in );
And std::string
has no implicit conversion to c-style string, but explicit one: c_str()
.
Use:
...
ifstream myfile ( file.c_str() );
...
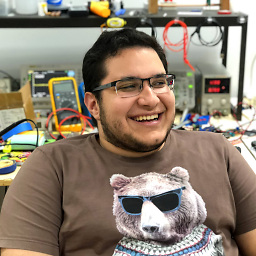
Author by
Nathan Campos
Electrical Engineer, Ham radio operator, photographer, used to be a programmer.
Updated on June 07, 2022Comments
-
Nathan Campos 7 months
I'm learning C++ and i'm getting some troubles when i'm trying to use a String in a ifstream method, like this:
string filename; cout << "Enter the name of the file: "; cin >> filename; ifstream file ( filename );
Here is the full code:
// obtaining file size #include <iostream> #include <fstream> using namespace std; int main ( int argc, char** argv ) { string file; long begin,end; cout << "Enter the name of the file: "; cin >> file; ifstream myfile ( file ); begin = myfile.tellg(); myfile.seekg (0, ios::end); end = myfile.tellg(); myfile.close(); cout << "File size is: " << (end-begin) << " Bytes.\n"; return 0; }
And here is the error of the Eclipse, the x before the method:
no matching function for call to `std::basic_ifstream<char, std::char_traits<char> >::basic_ifstream(std::string&)'
But when i try to compile in Eclipse it put an x before the method, that indicates an error in the syntax, but what is wrong in the syntax? Thanks!