Ignore milliseconds when comparing two datetimes
Solution 1
Create a new DateTime value with the milliseconds component set to 0:
dt = dt.AddMilliseconds(-dt.Millisecond);
Solution 2
I recommend you use an extension method:
public static DateTime TrimMilliseconds(this DateTime dt)
{
return new DateTime(dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, 0, dt.Kind);
}
then its just:
if (dtOrig.TrimMilliseconds() == dtNew.TrimMilliseconds())
Solution 3
Care should be taken, if dt
has non-zero microseconds (fractions of millis). Setting only milliseconds to zero is not enough.
To set millis and below to zero (and get a succesfull comparison), the code would be:
dt = dt.AddTicks(-dt.Ticks % TimeSpan.TicksPerSecond); // TimeSpan.TicksPerSecond=10000000
Solution 4
TimeSpan difference = dtNew - dtOrig;
if (difference >= TimeSpan.FromSeconds(1))
{
...
}
Solution 5
You can subtract them, to get a TimeSpan
.
Then use TimeSpan.totalSeconds()
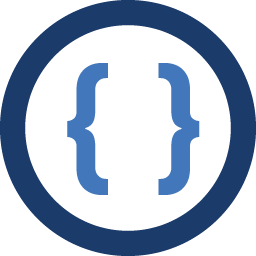
Admin
Updated on October 16, 2021Comments
-
Admin over 2 years
This is probably a dumb question, but I cannot seem to figure it out. I am comparing the LastWriteTime of two files, however it is always failing because the file I downloaded off the net always has milliseconds set at 0, and my original file has an actual value. Is there a simple way to ignore the milliseconds when comparing?
Here's my function:
//compare file's dates public bool CompareByModifiedDate(string strOrigFile, string strDownloadedFile) { DateTime dtOrig = File.GetLastWriteTime(strOrigFile); DateTime dtNew = File.GetLastWriteTime(strDownloadedFile); if (dtOrig == dtNew) return true; else return false; }
Thanks in advance
-
Ash Burlaczenko almost 13 yearsSo your method returns a DateTime but is defined as returning a string.
-
TheCloudlessSky over 10 yearsWarning: this won't work when the
DateTime
has non-zero microseconds. See @PeterIvan's or @DeanChalk's answers. -
Mickey Mouse over 9 yearsI don't know why all programmers needs too many lines when rteurning a bool function with
-
Luke Hutton over 9 yearsPerhaps preserve the
DateTimeKind
as well by addingdt.Kind
to end of ctor -
Purusartha over 9 yearswhere did you get ZeroMilliseconds() from?
-
Adamy almost 9 yearsreturn new DateTime(dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, 0, dt.Kind);
-
Simon MᶜKenzie over 8 yearsRun your code in the UK, Europe or Australia and see what happens. I'm not a fan of this approact anyway, but you need to be using an invariant culture or
DateTime.ParseExact
for this to be even remotely reliable. -
Larry over 8 yearsThis is the way to go IMHO.
-
Jeppe Stig Nielsen almost 8 yearsThis is the best solution. Fast, and preserves
dt.Kind
. I know it gives the same in all cases, but to me it is more natural to usedt = dt.AddTicks(-(dt.Ticks % TimeSpan.TicksPerSecond));
, i.e. use%
operator first, and then negate. That is because the behavior of%
with a negative first operand seems a bit confusing to me, so I prefer my version where%
operates on two positive operands. -
Jeppe Stig Nielsen almost 8 yearsWith Peter Ivan's solution, it is natural to just do
public static DateTime TruncateTo(this DateTime dt, long truncateResolution) { return dt.AddTicks(-(dt.Ticks % truncateResolution)); }
. Much shorter and clearer. Then use like this:DateTime dtSecs = DateTime.Now.TruncateTo(TimeSpan.TicksPerSecond); DateTime dtHrs = DateTime.Now.TruncateTo(TimeSpan.TicksPerHour);
and so on. You can even truncate to more strange resolutions, like multiples of 3 hours (3 * TimeSpan.TicksPerHour
). -
Peter Ivan almost 8 years@JeppeStigNielsen: Your code is exactly the same as mine. According to operator precedence, you multiply and/or divide and just then add/negate. So the parentheses change nothing, just clarify the well-established logic.
-
Jeppe Stig Nielsen almost 8 yearsNo that is incorrect. In rare cases (that cannot occur in your use above, so your use is fine), there is a difference. Consider this code (where
a
andb
must not be declaredconst
):long a = long.MinValue; long b = 10L; long x = (-a) % b; long y = -(a % b); long z = -a % b;
Here the value ofx
will be negative, because negation ofa
givesa
again, and a negative value%
-ed on a positive value gives a negative value. But the value ofy
is positive, because this time we negate a negative result between-10
and0
, and that gives a positive number. Now checkz
to see it! -
Doug Domeny almost 4 yearsThe dt.Kind should be set for each new DateTime
-
Doug Domeny almost 4 yearsThe value.Kind should be set in new DateTime