In a batch file, how would I check if today's date is after a set date?
12,177
Solution 1
In order to compare the dates you need to strip out the individual components of the current date and then put them back together into a comparable format (YYYY-MM-DD
):
@ECHO OFF
SET FirstDate=2015-01-24
REM These indexes assume %DATE% is in format:
REM Abr MM/DD/YYYY - ex. Sun 01/25/2015
SET TodayYear=%DATE:~10,4%
SET TodayMonth=%DATE:~4,2%
SET TodayDay=%DATE:~7,2%
REM Construct today's date to be in the same format as the FirstDate.
REM Since the format is a comparable string, it will evaluate date orders.
IF %TodayYear%-%TodayMonth%-%TodayDay% GTR %FirstDate% (
ECHO Today is after the first date.
) ELSE (
ECHO Today is on or before the first date.
)
Solution 2
@if (@X)==(@Y) @end /* JScript comment
@echo off
set "comp_date=2015/1/20"
rem :: the first argument is the script name as it will be used for proper help message
for /f %%# in ('cscript //E:JScript //nologo "%~f0" "%comp_date%"') do set comp=%%#
if %comp% EQU -1 (
echo current date is bigger than %comp_date%
) else (
echo current date is less than %comp_date%
)
exit /b %errorlevel%
@if (@X)==(@Y) @end JScript comment */
var ARGS = WScript.Arguments;
var compdate=ARGS.Item(0);
var c_date=(new Date()).getTime();
var comp_date=(new Date(compdate)).getTime();
//WScript.Echo(c_date);
//WScript.Echo(comp_date);
WScript.Echo(comp_date<c_date);
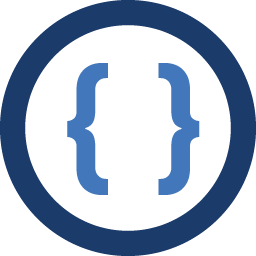
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm trying to check if today's date is after a certain date so that I can make a batch file that will only run after a certain date.
Right now I have:
@ECHO off IF %date% GTR 24/01/2015( ECHO it is after 24/01/2015 ) pause
But that doesn't work.
-
TT. over 6 yearsI tried this one, didn't work real well. I did get it to work however with taking the right 10 characters of
%DATE%
first, then doing the year, month and day stripping. So firstSET f=%DATE%
, thenSET dt=%f:~-10%
and then working ondt
to strip the year, month and day fields.