Include assets when building angular library
Solution 1
As already said, angular library now support assets since the v9.x of angular.
But it isn't well explained on their website. To make it work you'll have to:
- Add an assets folder at the root of your library project
- Add
"assets": ["./assets"],
into theng-package.json
file of the library
{
"$schema": "../../node_modules/ng-packagr/ng-package.schema.json",
"dest": "../../dist/icon",
"assets": ["./assets"], // <-- Add it here
"lib": {
"entryFile": "src/public-api.ts"
}
}
Reminder: tsconfig.lib.json path do not work in an angular libraries, so after your change you may have to edit manually the import of the assets with relative path
Then you could just add what you want in this folder
Additional tips : Use it within your project
Then if you wish to use it into the project it get imported into, do :
Assets, js, styles in angular.json
Add those files into your angular.json
file
{
/*...*/
"assets": [ // Import every assets
{
"glob": "**/*",
"input": "./node_modules/custom-project/assets",
"output": "/assets/"
}
],
"styles" : [ // Only custom css
"node_modules/custom-project/assets/my-css-file.css"
],
"scripts" : [
"node_modules/custom-project/assets/my-js-file.js"
]
}
Js as part of the app.module
You could directly also add js into children file even if I'm not sure if it really lazy loading those files.
example, into your home.module.ts
file, import
import 'custom-project/assets/my-js-file.js'
Solution 2
Like you said in your answer, it wasn't possible to force the packager to pack assets along with the build files.
Now, ng-packagr released a new version that allows you to include the assets along with the build files:
You can copy these assets by using the assets option.
Edit the ng-package.json
file in your library (or projects/<your-lib>/ng-package.json
file if you use multi-project workspace) to define the assets which should be included:
{
"ngPackage": {
"assets": [
"CHANGELOG.md",
"./styles/**/*.theme.scss"
],
"lib": {
...
}
}
}
More information here: https://github.com/ng-packagr/ng-packagr/blob/master/docs/copy-assets.md
Solution 3
Looking at other posts and issues on github, it looks like there is no way to force the packager to pack assets along with the build files. What I ended up doing instead is copying the assets
folder to the dist
folder manually before deploying it to npm. After that I would just have to tell users to manually @import
the icon_font.css
into one of their stylesheets.
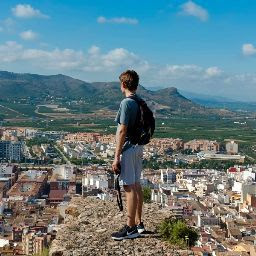
Aivaras Kriksciunas
Updated on September 17, 2021Comments
-
Aivaras Kriksciunas over 2 years
I am building a library which contains a custom icon font. However, when I build my library using
ng build <library-name> --prod
, the assets folder is not included in the build, which means icons do not show up when using the production build.I've tried multiple solutions like adding the
assets
array inangular.json
and copying the assets folder to the created dist folder.I am using Angular 8, and the library was created using angular-cli.
I tried including the fonts two ways: using
@import url( '../assets/icon_font.css' );
in one of the style files and adding../assets/icon_font.css
to styleUrls in one of the components that require it. (icon_font.css
is the css file that includes the icon fonts)Here's the layout of my library directory:
- src - lib - assets - icon_font.css - font files - component that requires icons - style sheet that has @import icon_font.css - other components and classes
I would like the .ttf and other font files in the
assets/
directory to be available in the exporteddist
folder. -
Mehul Parmar almost 4 yearsWhat is the source path you mentioned in your library code to access assets which were present in your library? Where did you copy assets exactly in the dist folder? Can you help?
-
theMayer over 3 yearsThis answer would be a lot more useful if it provided the context for these lines. What file? Where in the file?
-
Shishibi over 3 yearsthere should be a file ng-package.json in your angular library. It's information for your packager
-
Eric over 3 yearsIs it possible to copy assets from the node_modules folder of the library project? I have tried "assets": ["../../node_modules/@foo/core/assets"] but no luck. My hope was that it would create an "assets" folder under dist/<project> and copy all the assets from foo. This works with a normal Angular project by adding the assets configuration to the build/builder/options in the angular.json for angular-devkit/build-angular:browser.
-
Nirmal over 3 years@rbalet Looks like post Angular 6, using the syntax you have given, you cannot add assets from a node module if they are not in the src directory. Instead of the string path, you have to put this object in the assets array:
{ "glob": "**/*", "input": "./node_modules/module_name/assets", "output": "./assets" }
-
Raphaël Balet over 3 years@Nirmal,Thanks I've updated the answer. @Eric, should work now, don't forget to also add it under the assets found under
production
-
Dani P. over 3 yearsThe solution works but in an Angular library created with the CLI the assets folder is inside the src folder, not at the same level. I don't get the point of having a solution for copying assets in Angular that is not compliant with default folder structure. It's not your fault of course, just saying that this seems to be a poor design decision by Angular or ng-package team.
-
German Quinteros over 2 yearsThanks to @yntelectual for including where we should apply the fix.
-
Noy Oliel over 2 years@Raphaël Balet This works but then I get an error problem with the import statements for of the scss style files. How can this be fixed? I tried adding to tsconfig.lib.json -> compilerOptions -> paths the following: "assets": ["./assets"] and also tried "assets/*": ["./assets/*"] but non works
-
Raphaël Balet over 2 years@olNoy Libraries can not work with the path of the
tsconfig.lib.json
the problem is that only the main project is taken, so you have to do it as I did, meaning moving theassets
folder under the project directly. So you'll have to follow my instruction and do exactly like it if you want to make it work. Or I may misunderstand what you did try to achieve -
Noy Oliel over 2 years@Raphaël Balet, I did "exactly" as described above and as you wrote, it works, however since assets folder was moved to a different location (by following your solution) I started having problems with the non relative paths, such as:@import "assets/somefolder/somefile" as they are not recognizing the files. only relative paths work (e.g. ../../assets/ect) To fix issue I tried playing with tsconfig.lib.json, however this failed. I was wondering what can I do in order to fix this issue as the assets folder is not in the original location.
-
Raphaël Balet over 2 years@olNoy Ah, now I see the problem. Can you try the following. Let your
assets
folder where it was, change theng-package.json
and target yourassets
folder like following ->"assets": ["./[yourPath]/assets"],
? You shall not use tsconfig.lib.json path in angular libraries. I'm not sure it will work. I case this do not work, then you'll have to correct the assets path. Another mention, if you're using a lot of scss mixins, then you would maybe like to add them to another library, so you could just@use '~@myLib/my-scss-mixin-lib' as *
-
Aamer Shahzad over 2 yearsThis feature is added in ng-packager v9.x and higher. for the lower version, you have to copy manually.
-
Hasan Can Saral about 2 yearsHow do you import
theme.scss
afterwards in the child projects?