Inline-styles in ReactJS
Solution 1
Declare your inline style as an object:
<button style={{ background: 'yellow' }} onClick={this.handleClick}>
{this.state.isHelpOn ? 'HELP ON' : 'HELP OFF'}
</button>
Solution 2
You're missing a set of brackets and some quotes:
<button style={{background: 'yellow'}} onClick={this.handleClick}>
{this.state.isHelpOn ? 'HELP ON' : 'HELP OFF'}
</button>
React's style prop expects an object ({background: 'yellow'}
) and since it's not a simple string, you need the extra set of brackets around the prop {{...}}
.
Solution 3
First of all style has to be passed as an object.
Secondly - css value has to be a string.
style={{ background: 'yellow' }}
Solution 4
You need to wrap it in another {}
:
<button style={{background:'yellow'}} onClick={this.handleClick}>
{this.state.isHelpOn ? 'HELP ON' : 'HELP OFF'}
</button>
The outer set of {}
s just tells the JSX compiler that what is inside is JavaScript code. The inner set of {}
s creates a JavaScript object literal.
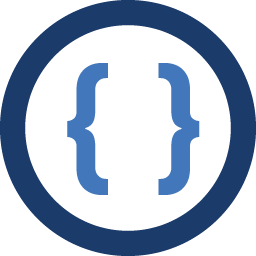
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
I'm new to ReactJS. I was trying to change simultaneously the text and the colour of a button when clicking on it. This code works:
class ToggleHelp extends React.Component { constructor(props) { super(props); this.state = {isHelpOn: true}; // This binding is necessary to make `this` work in the callback this.handleClick = this.handleClick.bind(this); } handleClick() { this.setState(prevState => ({ isHelpOn: !prevState.isHelpOn })); } render() { return ( <button onClick={this.handleClick}> {this.state.isHelpOn ? 'HELP ON' : 'HELP OFF'} </button> ); } } ReactDOM.render( <ToggleHelp />, document.getElementById('root') );
But when I try to aplly an inline style like the following, code stops working.
<button style={background:yellow} onClick={this.handleClick}> {this.state.isHelpOn ? 'HELP ON' : 'HELP OFF'} </button>
I've tried several times, doing it in various ways. I'd like it to be an inline style for the moment. Is it possible to apply inline styles directly from React? If yes, the idea is to evaluate the status and set one color on another via conditional statement.