Invalid Hash key in facebook app in android
Solution 1
You are getting the hash-key with debug key... Which may work if you haven't sign the package and running app in debug mode. What you need to do is :
1) Go to the manifest file and add to the application android:debuggable="true".
2) Sign the application, capy and install to your device manually or for use "adb install path_to_apk" from command line.
3) Now run your app and monitor the logcat.
4) You will get printed a new key which will be the matching key with key facebook app is showing in the error msg, The key you have got is now having a = sign in the last.
5) Register this key on facebook developer site
Alternate Trick
You can do one other thing Simply register key which is showing in the error msg "The key hash ### does not match any stored key hashes" Just add the = in the end of the ###. It will be like ###=
you are done!! Hope this will work.
Solution 2
Add this function into your class,and call this function in oncreate methode,then generate sign apk and run sign apk in your device and check log-cat, copy generated hash key to facebook developer console.
private void showHashKey()
{
// Add code to print out the key hash
try {
PackageInfo info = getPackageManager().getPackageInfo(
"com.kisan.kisan",
PackageManager.GET_SIGNATURES);
for (Signature signature : info.signatures) {
MessageDigest md = MessageDigest.getInstance("SHA");
md.update(signature.toByteArray());
Log.d("KeyHash:", Base64.encodeToString(md.digest(), Base64.DEFAULT));
}
} catch (PackageManager.NameNotFoundException e) {
} catch (NoSuchAlgorithmException e) {
}
}
Check facebook authentication,it will work fine
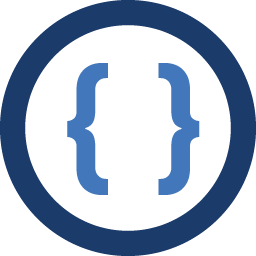
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
I got hash key from below code in android:
try { PackageInfo packageInfo = getPackageManager().getPackageInfo(getPackageName(), PackageManager.GET_SIGNATURES); for (Signature signature : packageInfo.signatures) { MessageDigest md = MessageDigest.getInstance("SHA"); md.update(signature.toByteArray()); Log.d("KeyHash:", Base64.encodeToString(md.digest(), Base64.DEFAULT)); } } catch (NameNotFoundException e1) { Log.e("Name not found", e1.toString()); } catch (NoSuchAlgorithmException e) { Log.e("No such an algorithm", e.toString()); } catch (Exception e){ Log.e("Exception", e.toString()); }
But it was invalid key... Please suggestion how to verify hash key for Facebook login....
-
Arun Badole over 9 yearsThanks...I tried by generating my own hash key for signed version, but this didn't work. So I tried alternate trick.. Worked:)