Is it possible to set a maximum value for a column in SQL Server 2008 r2
Solution 1
A trigger. I tested this.
CREATE TRIGGER dbo.Table_2update
ON dbo.Table_2
FOR INSERT, UPDATE
AS BEGIN
UPDATE dbo.Table_2 SET dbo.Table_2.memberID = 10000
FROM INSERTED
WHERE inserted.id = Table_2.id
AND dbo.Table_2.memberID > 10000
END
Solution 2
If you want to set a maximum value on a column of a SQL table, one thing you can do is add a CHECK
constraint with specific criteria for that column:
ALTER TABLE dbo.mytable ADD CONSTRAINT CK_HAPPINESS_MAX CHECK (PokemonHappiness <= 10000)
However, this won't handle out-of-bounds input in a graceful fashion; if your input violates the CHECK
constraint, SQL will simply throw an error.
To properly handle this sort of input, you should probably use a CASE
expression as others suggest, and maybe use a CHECK
constraint as a hard bound to what can be inserted (just in case of unmoderated input values).
Solution 3
When you want to set it to 10000 then don't set it to 10040. Your "auto-update" would have side-effect and would be very error-prone(consider that you'll forget it or someone doesn't know it). But you could use a CASE
:
UPDATE dbo.MyTable
SET PokemonHappiness =
( CASE
WHEN (PokemonHappiness + 50) > 10000 THEN 10000
ELSE (PokemonHappiness + 50)
END
)
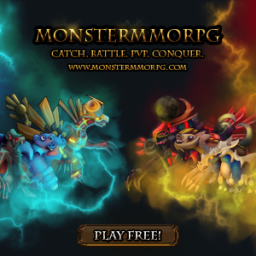
MonsterMMORPG
Hello. I am the only owner and developer of web based online MMORPG game MonsterMMORPG. I am a computer engineer from Turkey and i am currently doing MA at computer engineering. I am specialized with C# & ASP.net. http://www.monstermmorpg.com/ MonsterMMORPG is a Free To Play Browser Based Online Monster MMORPG Game Better Than Online Pokemon Games You will love it's awesome Monsters We have many different unique features. So i suggest you to check them out. Our game is similiar with Pokemon games but it is unique. Like diablo and torch light. You should visit following sites related to us MonsterMMORPG Facebook Pokemon Games Lovers Facebook Application MonsterMMORPG Youtube Channel Monster Game Forum Canavar Oyunu Forum Pokemon Fakemon DeviantArt MonsterMMORPG Google Plus MonsterMMORPG Twitter MonsterMMORPG Review On Browsergamez MonsterMMORPG Review On mmohuts MonsterMMORPG Developer Blog At MMORPG.com MonsterMMORPG Review On onrpg MonsterMMORPG On GameSpot MonsterMMORPG Wiki MonsterMMORPG On 1UP MonsterMMORPG Digg MonsterMMORPG Official Google Plus Page
Updated on November 23, 2022Comments
-
MonsterMMORPG 6 months
My question is pretty simple but I'm not sure if it's possible.
Assume I have a table and it contains the column below
PokemonHappiness smallint
Is it possible to set a maximum value for that column?
For instance if I set the maximum to 10000 and send a query like this
--Assume that PokemonHappiness is equal to 9990 update mytable set PokemonHappiness = PokemonHappiness+50
it should become 10000 instead of 10040
Is this possible?
-
Tim Schmelter over 10 yearsWhen you want to set it to 10000 then don't set it to 10040.
-
-
MonsterMMORPG over 10 yearsi know i can use case. but that requires modification to my all queries. that is why i am asking sql server side possible or not.
-
MonsterMMORPG over 10 yearswould this check constraint cause any performance issue ? thanks.
-
Tim Schmelter over 10 years@MonsterMMORPG: You could use a trigger for this, but i wouldn't recommend it because triggers have cost and they are "magic" (what i've mentioned above).
-
mikurski over 10 yearsAny kind of additional check or process has the potential to impact performance. Given that the specified check only compares two integer values, I'd say that the impact would be pretty minimal on a per-row basis.