Is std::vector memory freed upon a clear?
Solution 1
The memory remains attached to the vector. That isn't just likely either. It's required. In particular, if you were to add elements to the vector again after calling clear()
, the vector must not reallocate until you add more elements than the 1000 is it was previously sized to.
If you want to free the memory, the usual is to swap with an empty vector. C++11 also adds a shrink_to_fit
member function that's intended to provide roughly the same capability more directly, but it's non-binding (in other words, it's likely to release extra memory, but still not truly required to do so).
Solution 2
The vector's memory is not guaranteed to be cleared. You cannot safely access the elements after a clear. To make sure the memory is deallocated Scott Meyers advised to do this:
vector<myStruct>().swap( vecs );
Cplusplus.com has the following to say on this:
Removes all elements from the vector, calling their respective destructors, leaving the container with a size of 0.
The vector capacity does not change, and no reallocations happen due to calling this function. A typical alternative that forces a reallocation is to use swap:...
Solution 3
The destructor is called on the objects, but the memory remains allocated.
Solution 4
No, memory are not freed.
In C++11, you can use the shrink_to_fit
method for force the vector to free memory.
http://www.cplusplus.com/reference/vector/vector/
Solution 5
.resize(0)
and .clear()
don't release or reallocate allocated memory, they just resize vector to zero size, leaving capacity same.
If we need to clear with freeing (releasing) memory following works:
v = std::vector<T>();
It calls &&
overload of =
operator, which does moving, similar behaviour as with swap()
solution.
This assignment technique works when there is &&
overload of =
operator, and it'is guaranteed to be present in all C++11 STD libraries, see here. So if you compile with -std=c++11
(gcc/clang) or /std:c++11
(msvc) switch (or later) my solution is guaranteed to work.
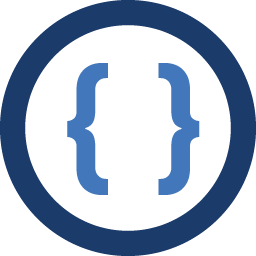
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
Suppose I have a std::vector of structs. What happens to the memory if the vector is clear()'d?
std::vector<myStruct> vecs; vecs.resize(10000); vecs.clear();
Will the memory be freed, or still attached to the vecs variable as a reusable buffer?