Is there a way to store java variable/object in application context without xml or properties file
If you create a class and put @Configuration
annotation over it, and declare some beans with @Bean
annotation, they become application managed beans.
@Configuration
public class ConfigurationClass {
@Bean("myString")
public String getString() {
return "Hello World";
}
}
Then anywhere from your code you can call AnnotationConfigWebApplicationContext.getBean("myString")
to get your bean.
Now there could be concurrency issues (as mentioned by davidxxx ). For that you can use SimpleThreadScope
. Look at this link to learn how to register it with application context and where to place that annotation (or google it).
Or if you don't want to deal with that, then a @Scope("request")
should help. The bean with @Scope("request")
would be created for every new incoming request thus it’s thread safety is guaranteed since it’s created every time a new request comes in.
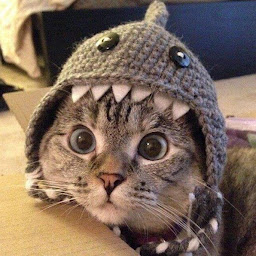
anu l
Updated on June 05, 2022Comments
-
anu l about 2 years
I want to store a particular variable (String or Object) in application context in spring boot application. But I don't want to use an xml or properties file for storing it.
There will be a function which will store the data in application context. I should be able to retrieve it, modify it, delete it or add more data.
Basically I want to store data in application context after its initialization has been done.
-
davidxxx about 7 yearsHello :) Yes they become. The problem is the concurrency : the op wants to store/modify/get data from it.
-
davidxxx about 7 years@Faraz Durrani We could believe but I don't think as
@Scope(value = "request")
will create a distinct instance by HTTP request. If you are interested in this matter, you can look at the accepted answer here: stackoverflow.com/questions/15745140/…