Is there any way to generate a random number within a given range using only CSS?
15,710
Solution 1
There is currently no way to do this in pure CSS, however if you're using a CSS pre-processor, such as LESS, then you can do the following:
@randomMargin: `Math.round(Math.random() * 100)`;
div {
margin-left: ~'@{randomMargin}px';
}
The reason this works is because LESS will evaluate JavaScript expressions.
If you want to break it into a random mixin/function, you could use:
.random(@min, @max) {
@random: `Math.round(Math.random() * (@{max} - @{min}) + @{min})`;
}
div {
.random(-100, 100);
margin-left: ~'@{random}px';
}
Which will compile with a different margin-left
value each time:
div {
margin-left: 18px;
}
However, I am not sure how practical this would be in a production environment since your CSS should already be compiled/minified rather than compiled on the fly. Therefore you should just use straight JavaScript in order to achieve this.
Solution 2
Dont think CSS has that capability but LESS will help
http://csspre.com/random-numbers/
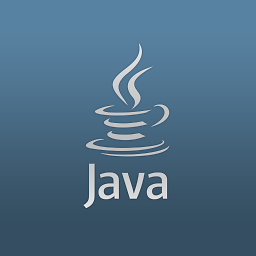
Author by
Alexcamostyle
Updated on June 04, 2022Comments
-
Alexcamostyle 12 months
For example, something like:
div { margin-left: random(-100, 100); }