React.js generate new random number
getInitialState
returns an object. You're just calling this.getInitialState()
and throwing away the object. To update the state you need to call this.setState
fail: function(){
this.setState(this.getInitialState());
},
There's no magic wrapper around getInitialState, the function just does what you tell it to do, and it's used by react when instancing your component.
I removed this.setState({score: 0})
because it's provided by your getInitialState.
Also, ButtonContainer should be passing the answer up, not updating its props and passing itself to the onClick callback. If you're reading props other than your own, something is wrong.
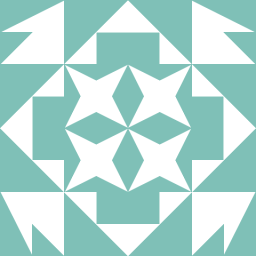
Boyswan
Updated on June 04, 2022Comments
-
Boyswan about 1 year
I'm trying to create a new random number which will be set as state after a method. It works perfectly on refresh, but I can't 'generate' a new number after the button click.
Here's my code at the moment:
var QuizContainer = React.createClass({ randomNumber: function(){ return Math.floor((Math.random() * Data.length) + 0); }, getInitialState: function(){ var rand = this.randomNumber(); return { answerList: Data[rand].answer, answerQuestion: Data[rand].question, correctAnswer: Data[rand].correct, score: 0, timer: 30 } }, success: function(){ this.setState({score: this.state.score + 1}) }, fail: function(){ this.setState({score: 0}) this.getInitialState(); }, handleClick: function(child){ child.props.singleAnswer == this.state.correctAnswer ? this.success() : this.fail() }, render: function(){ return( <div> <Timer timer={this.state.timer} /> <Score currentScore={this.state.score} /> <QuestionContainer answerQuestion={this.state.answerQuestion} /> <ButtonContainer onClick={this.handleClick} correctAnswer={this.state.correctAnswer} answerList={this.state.answerList} /> </div> ) } }) module.exports = QuizContainer;
If anyone's able to help I'd be very grateful! Thanks!