Javascript: url containing random number
Solution 1
I would add a parameter but you can leave it out if needed:
var url = "http://www.mypage.com/index.php?rnd="+Math.random()
or
var url = "http://www.mypage.com/index.php?rnd="+new Date().getTime()
Link:
<a href="http://www.mypage.com/index.php?rnd=1" onClick="this.href=this.href.split('?')[0]+'?rnd='+new Date().getTime()">Mostly random</a>
Note that if you have more than one assignment - for example in a loop, you need to add to the getTime since an iteration of the loop is faster than a millisecond:
var rnd = new Date().getTime();
for (var i=0;i<links.length;i++) {
links[i].href = "http://www.mypage.com/index.php?rnd="+(rnd+i);
}
UPDATE to use the URL constructor with searchParams
const addRnd = urls => {
let rnd = new Date().getTime();
return urls.map((urlStr,i) => {
let url = new URL(urlStr);
url.searchParams.set("rnd",rnd+i); // in case called multiple times
return url;
});
};
const urls = addRnd( ["http://www.mypage.com/index1.php","http://www.mypage.com/index2.php","http://www.mypage.com/index3.php"])
console.log(urls)
Solution 2
<a href="http://www.mypage.com/index.php?" onclick="this.href+=new Date().getTime();return true;">link</a>
Solution 3
var lower = 0;
var upper = 100000000;
var url = "http://www.mypage.com/index.php?"+(Math.floor(Math.random()*(upper-lower))+lower)
it generates a random X from 0(lower) to 100000000 (upper), you can obv set the bounds you want ;)
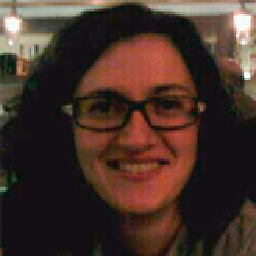
ADM
Programming enthusiast, delighted to have been born in this era. At this moment I’m looking to work remotely so I can continue giving the best of my work in the moments of better productivity within the connectivity of team work
Updated on April 14, 2020Comments
-
ADM over 3 years
A friend is linking my page from his site. As I need users to avoid caching when visiting my page, I want the url to have this form:
http://www.mypage.com/index.php?456646556
Where 456646556 is random number.
As my friend does not have installed php, how can I build the link with the random number using Javascript?
Also my friend asked me to give him just the url, with no further functions as his page is already loaded with them. Can it be done?
Thanks a lot
-
mplungjan over 12 yearsdocument.location is deprecated for document.URL, window.location is what you want here
-
Ivo Wetzel over 12 years@mplungjan Just woke up, fixed it :)
-
mplungjan over 12 yearsIt will keep adding a new date each time it is clicked. That is why I did a split in my suggestion
-
igor about 12 yearsI thought it's ok for situation with just couple of clicks. If you expect someone to hammer this link, then you have to use split.