Is (*x).y the same as x->y?
Solution 1
In C, c->m
is equivalent to (*c).m
. The parentheses are necessary since .
has a higher precedence than *
. Any respectable compiler will generate the same code.
In C++, unless ->
or *
is overloaded, the equivalence is as above.
Solution 2
There are 3 operators here, *
, .
and ->
. This is important, because .
and ->
both have precedence 1, but *
has precedence 2. Therefore *foo.bar
is not the same as foo->bar
and parenthesis are required, like in (*foo).bar
.
All are original C operators and have been around forever.
Solution 3
In C, a->b
and (*a).b
are 100% equivalent, and the very reason for introduction of ->
into C was precedence—so that you don't have to type the parentheses in (*a)
.
In C++, operator *
and operator ->
can be overridden independently, so you can no longer say that a->b
and (*a).b
are equivalent in all cases. They are, however, 100% equivalent when a
is of a built-in pointer type.
Solution 4
Operator ->
is standard in C. Both .
and ->
allow to access a struct
field. You should use .
on a struct
variable and ->
on a struct pointer
variable.
struct foo {
int x;
int y;
}
struct foo f1;
f1.x = 1;
f1.y = 3;
struct foo *f2 = &f1;
printf("%d\n", f1.x); // 1
printf("%d\n", f2->x); // 1
The *
operator is called dereference operator
and returns the value at the pointer address. So (*f2).x
is equivalent to f2->x
.
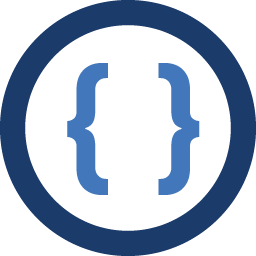
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
Is operator
->
allowed to use in C instead of.
? Does its availability depend on compiler we are using? Is->
operator available in the last C standard or does it come from the C++ standard? How those two differ?