isset PHP isset($_GET['something']) ? $_GET['something'] : ''
Solution 1
It's commonly referred to as 'shorthand' or the Ternary Operator.
$test = isset($_GET['something']) ? $_GET['something'] : '';
means
if(isset($_GET['something'])) {
$test = $_GET['something'];
} else {
$test = '';
}
To break it down:
$test = ... // assign variable
isset(...) // test
? ... // if test is true, do ... (equivalent to if)
: ... // otherwise... (equivalent to else)
Or...
// test --v
if(isset(...)) { // if test is true, do ... (equivalent to ?)
$test = // assign variable
} else { // otherwise... (equivalent to :)
Solution 2
In PHP 7 you can write it even shorter:
$age = $_GET['age'] ?? 27;
This means that the $age
variable will be set to the age
parameter if it is provided in the URL, or it will default to 27.
See all new features of PHP 7.
Solution 3
That's called a ternary operator and it's mainly used in place of an if-else statement.
In the example you gave it can be used to retrieve a value from an array given isset returns true
isset($_GET['something']) ? $_GET['something'] : ''
is equivalent to
if (isset($_GET['something'])) {
echo "Your error message!";
} else {
$test = $_GET['something'];
}
echo $test;
Of course it's not much use unless you assign it to something, and possibly even assign a default value for a user submitted value.
$username = isset($_GET['username']) ? $_GET['username'] : 'anonymous'
Solution 4
You have encountered the ternary operator. It's purpose is that of a basic if-else statement. The following pieces of code do the same thing.
Ternary:
$something = isset($_GET['something']) ? $_GET['something'] : "failed";
If-else:
if (isset($_GET['something'])) {
$something = $_GET['something'];
} else {
$something = "failed";
}
Solution 5
It is called the ternary operator. It is shorthand for an if-else block. See here for an example http://www.php.net/manual/en/language.operators.comparison.php#language.operators.comparison.ternary
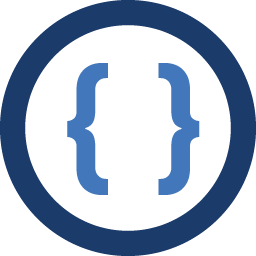
Admin
Updated on August 22, 2021Comments
-
Admin almost 3 years
I am looking to expand on my PHP knowledge, and I came across something I am not sure what it is or how to even search for it. I am looking at php.net isset code, and I see
isset($_GET['something']) ? $_GET['something'] : ''
I understand normal isset operations, such as
if(isset($_GET['something']){ If something is exists, then it is set and we will do something }
but I don't understand the ?, repeating the get again, the : or the ''. Can someone help break this down for me or at least point me in the right direction? -
Bright umor almost 4 yearsThis isn't really plain.