Java 2D: Moving a point P a certain distance closer to another point?
Solution 1
Vectors to the rescue!
Given points A and B. Create a vector V from A to B (by doing B-A). Normalize vector V into a unit vector and then just multiply it with the distance, d, you want and finally add the resulting vector to point A. ie:
A_moved = A + |(B-A)|*d
Java(ish)
Vector2D a_moved = a.add(b.subtract(a).norm().multiply(d));
No angles, no nasty trig needed.
Solution 2
The shortest distance between two points is a line, so simply move that point x
units along the line that connects the two points.
Edit: I didn't want to give away the specifics of the answer if this is homework, but this is simple enough that it can be illustrated without being too spoiler-y.
Let us assume you have two points A
= (x1, y1) and B
= (x2, y2). The line that includes these two points has the equation
(x1, y1) + t · (x2 - x1, y2 - y1)
where t
is some parameter. Notice that when t = 1
, the point specified by the line is B
, and when t = 0
, the point specified by the line is A
.
Now, you would like to move B
to B'
, a point which is a new distance d
away from A
:
A B' B
(+)---------------------(+)-----------(+)
<========={ d }=========>
The point B'
, like any other point on the line, is also governed by the equation we showed earlier. But what value of t
do we use? Well, when t
is 1, the equation points to B
, which is |AB|
units away from A
. So the value of t
that specifies B'
is t
= d/|AB|
.
Solving for |AB| and plugging this into the above equation is left as an exercise to the reader.
Solution 3
You can minimize the difference along both axis by a percent (that depends on how much you want to move the points).
For example:
Point2D.Double p1, p2;
//p1 and p2 inits
// you don't use abs value and use the still point as the first one of the subtraction
double deltaX = p2.getX() - p1.getX();
double deltaY = p2.getY() - p1.getY();
// now you know how much far they are
double coeff = 0.5; //this coefficient can be tweaked to decice how much near the two points will be after the update.. 0.5 = 50% of the previous distance
p1.setLocation(p1.getX() + coeff*deltaX, p1.getY() + coeff*deltaY);
So you moved p1
halfway toward p2
. The good thing avoid abs
is that, if you choose which point will be moved and which one will stand still you can avoid if tests and just use the raw coefficient.
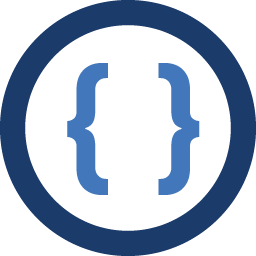
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
What is the best way to go about moving a Point2D.Double x distance closer to another Point2D.Double?
Edit: Tried to edit, but so went down for maintenance. No this is not homework
I need to move a plane (A) towards the end of a runway (C) and point it in the correct direction (angle a).
alt text http://img246.imageshack.us/img246/9707/planec.png
Here is what I have so far, but it seems messy, what is the usual way to go about doing something like this?
//coordinate = plane coordinate (Point2D.Double) //Distance = max distance the plane can travel in this frame Triangle triangle = new Triangle(coordinate, new Coordinate(coordinate.x, landingCoordinate.y), landingCoordinate); double angle = 0; //Above to the left if (coordinate.x <= landingCoordinate.x && coordinate.y <= landingCoordinate.y) { angle = triangle.getAngleC(); coordinate.rotate(angle, distance); angle = (Math.PI-angle); } //Above to the right else if (coordinate.x >= landingCoordinate.x && coordinate.y <= landingCoordinate.y) { angle = triangle.getAngleC(); coordinate.rotate(Math.PI-angle, distance); angle = (Math.PI*1.5-angle); } plane.setAngle(angle);
The triangle class can be found at http://pastebin.com/RtCB2kSZ
Bearing in mind the plane can be in in any position around the runway point