Java byte array compression
Solution 1
This will depend on the data you are compressing. For example if we take an array of 0
bytes it compresses well:
byte[] plain = new byte[10000];
byte[] compressed = compress(plain);
System.out.println(compressed.length); // 33
byte[] result = decompress(compressed);
System.out.println(result.length); // 10000
Solution 2
Compression always has overhead to allow for future decompression. If the compression produced no reduction in length (the data was unique or nearly unique) then the output file could be longer than the input file
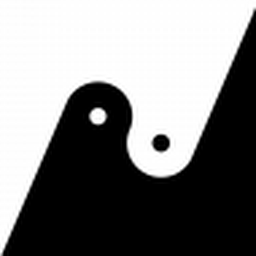
Lev Knoblock
I enjoy dabbling in projects related to cryptography, voxel rendering, physics simulation, and various other topics. Graduated with a BS in CSE from Michigan State University.
Updated on June 04, 2022Comments
-
Lev Knoblock almost 2 years
I'm trying to use the java
DeflaterOutputStream
andInflaterOutputStream
classes to compress a byte array, but both appear to not be working correctly. I assume I'm incorrectly implementing them.public static byte[] compress(byte[] in) { try { ByteArrayOutputStream out = new ByteArrayOutputStream(); DeflaterOutputStream defl = new DeflaterOutputStream(out); defl.write(in); defl.flush(); defl.close(); return out.toByteArray(); } catch (Exception e) { e.printStackTrace(); System.exit(150); return null; } } public static byte[] decompress(byte[] in) { try { ByteArrayOutputStream out = new ByteArrayOutputStream(); InflaterOutputStream infl = new InflaterOutputStream(out); infl.write(in); infl.flush(); infl.close(); return out.toByteArray(); } catch (Exception e) { e.printStackTrace(); System.exit(150); return null; } }
Here's the two methods I'm using to compress and decompress the byte array. Most implementations I've seen online use a fixed size buffer array for the decompression portion, but I'd prefer to avoid that if possible, because I'd need to make that buffer array have a size of one if I want to have any significant compression.
If anyone can explain to me what I'm doing wrong it would be appreciated. Also, to explain why I know these methods aren't working correctly: The "compressed" byte array that it outputs is always larger than the uncompressed one, no matter what size byte array I attempt to provide it.
-
Lev Knoblock almost 6 yearsAnd I completely failed to take that into account, considering I was testing it on random data. Makes sense now.
-
Karol Dowbecki almost 6 years@LevKnoblock makes sense, if the data was truly random it would be hard to compress. You do however want to use
try-with-resource
in your implementation to close the resources.