Java Convert this string to JSONArray
83,122
Solution 1
If you're talking about using the JSON in java library, then since your input string is a JSON object, not a JSON array, you should first load it using JSONObject:
String response=getResponseFromServer();
JSONObject jsonObject = new JSONObject(response);
After that, you can use toJSONArray() to convert a JSONObject to JSONArray given an array of key strings:
String[] names = JSONObject.getNames(jsonObject);
JSONArray jsonArray = jsonObject.toJSONArray(new JSONArray(names));
Solution 2
If you do a search right here on StackOverflow for Java String to JSONArray, you should get this answer: Converting from JSONArray to String then back again
JSONArray jArray;
String s = jArray.toString(); // basically what you have ;)
JSONArray newJArray = new JSONArray(s);
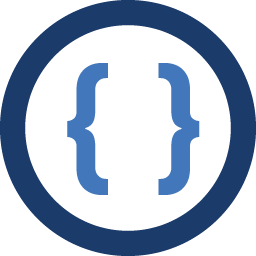
Author by
Admin
Updated on June 10, 2020Comments
-
Admin almost 4 years
I am getting the following
String
response from a server:{"fid":"1272","uri":"http://someurl/services/file/1272"}
I need to convert it into a
JSONArray
. Any help?By the way, I tried this and it does not work:
String response=getResponseFromServer(); JSONArray array = new JSONArray(response);
I get the error:
org.json.JSONException: Value {"fid":"1272","uri":"http://someurl/services/file/1272"} of type org.json.JSONObject cannot be converted to JSONArray