Java Generate Random Number Between Two Given Values
Solution 1
You could use e.g. r.nextInt(101)
For a more generic "in between two numbers" use:
Random r = new Random();
int low = 10;
int high = 100;
int result = r.nextInt(high-low) + low;
This gives you a random number in between 10 (inclusive) and 100 (exclusive)
Solution 2
Assuming the upper is the upper bound and lower is the lower bound, then you can make a random number, r, between the two bounds with:
int r = (int) (Math.random() * (upper - lower)) + lower;
Solution 3
int Random = (int)(Math.random()*100);
if You need to generate more than one value, then just use for loop for that
for (int i = 1; i <= 10 ; i++)
{
int Random = (int)(Math.random()*100);
System.out.println(Random);
}
If You want to specify a more decent range, like from 10 to 100 ( both are in the range )
so the code would be :
int Random =10 + (int)(Math.random()*(91));
/* int Random = (min.value ) + (int)(Math.random()* ( Max - Min + 1));
*Where min is the smallest value You want to be the smallest number possible to
generate and Max is the biggest possible number to generate*/
Solution 4
Like this,
Random random = new Random();
int randomNumber = random.nextInt(upperBound - lowerBound) + lowerBound;
Solution 5
Use Random.nextInt(int).
In your case it would look something like this:
a[i][j] = r.nextInt(101);
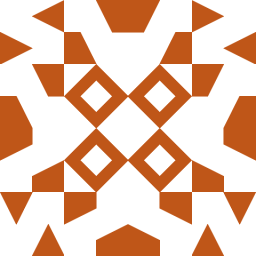
Mus
“Data Scientist (n.): Person who is better at statistics than any software engineer and better at software engineering than any statistician.” ― Josh Wills, Director of Data Engineering at Slack
Updated on October 31, 2020Comments
-
Mus over 2 years
I would like to know how to generate a random number between two given values.
I am able to generate a random number with the following:
Random r = new Random(); for(int i = 0; i < a.length; i++){ for(int j = 0; j < a[i].length; j++){ a[i][j] = r.nextInt(); } }
However, how can I generate a random number between 0 and 100 (inclusive)?
-
Mus about 12 yearsThank you - I have tried that but it does not seem to work. I am using the random numbers from 0 to 100 (inclusive) to populate a multidimensional array; when trying this it populates the array with extremely large and extremely small numbers. For example, -3.76556749E8 3.0207573E8 2.033182079E9 -6.86227134E8.
-
Erik about 12 yearsShow the rest of the code. What's e.g.
a
. -
Thomas about 12 yearsCould you post how you invoke
r.nextInt()
and with which values? -
itsbruce over 10 yearsBit verbose and clumsy. You shouldn't need to repeat that code, just to decide whether to have n1 or n2's value first.
-
SnakeDoc almost 9 years@Thomas
Random r = new Random(); System.out.println(r.nextInt(High - Low) + Low);
-
Stefan Schouten over 8 yearsMaybe a little late, and it's always bound to opinions and code styles, but usually you don't create a variable with an uppercase, so it's "better" to name it "random" instead of "Random". Classes are usually with uppercase :)
-
Krease about 8 yearsThis will fail in case where max is Integer.MAX_VALUE and min is 0. Use Math.round instead of casting to int to solve that.
-
Rizon over 6 yearsThis is exclusive to upper
-
Farruh Habibullaev over 6 yearsint r = (Math.random() * (upper - lower)) + lower; It would be inclusive for lower. It might be helpful.
-
Jens about 6 yearsWith respect to the Java naming convention the variable names should start with lower case character
-
Jens about 6 yearsWith respect to the Java naming convention the variable names should start with lower case character
-
Jens about 6 yearsWith respect to the Java naming convention the variable names should start with lower case character
-
Manjitha Teshara almost 5 yearsin this Result range is 10 to 100 ,r.nextInt(High-Low) given range is 0 to 90
-
JGFMK over 2 yearsDoes not work try 1 and 6 - for roll of dice. You guys are all giving wrong answers :
r.nextInt(high-low+1) + low;
-
Erik over 2 yearsAs described in the answer, the lower value is inclusive and the higher value is exclusive. So you'd need to use 1 and 7 for a dice roll.