Java Generics putting on Map<String, ? extends List<String>>
Solution 1
public void myMethod( Map<String, List<String>> map ) {
map.put("foo", Collections.singletonList("bar") );
}
You can't put a List
(the return type of Collections.singletonList()
into a Map
of ? extends List
since the actual type could be any implementation of List
. For example, it is not safe to put a generic List
into a Map<String,LinkedList>
since the List
might not be a LinkedList
. However, we can easily put a LinkedList
into a Map
of <String,List>
.
I think you were over thinking your generics. You do not have to use ? extends
for a non-generic class. For example, List<Object>
will hold any Object
, the ? extends
is not needed to add an object. A List<List<Foo>>
will only take List<Foo>
objects and not List<FooSubclass>
objects [Generic classes don't inherit based on their parameters]. This is where ? extends
comes into play. To add both List<Foo>
and List<FooSubclass>
to a List
, the type must be List<List<? extends Foo>>
.
Solution 2
Wouldn't Map<String, List<String>
work? Is there some particular reason you have to have a wildcard there at all?
Related videos on Youtube
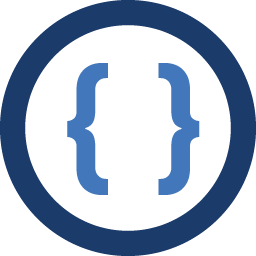
Admin
Updated on April 17, 2022Comments
-
Admin about 2 years
Is there a way to make the following implementation in a type safe manner?
public void myMethod( Map<String, ? extends List<String>> map ) { map.put("foo", Collections.singletonList("bar"); }
The above implementation doesn't work. It requires a
Map<String, ? super List<String>>
to compile the methodmap.put()
correctly. But myMethod won't accept any subtype of List this way. So, I have to useMap<String, ? extends List<String>>
instead. How can I solve this problem in a type safe manner? -
palantus almost 15 years+1 for a good explanation (now that your generics are visible :P).
-
KitsuneYMG almost 15 yearsOops. I keep thinking that since SOF filters text anyway that it fixes the angled bracket stuff. Oh well...