Java List generics syntax for primitive types
14,002
Solution 1
Use the wrapper class Byte
:
List<Byte> mylist = new ArrayList<Byte>();
Then, because of autoboxing, you can still have:
for (byte b : mylist) {
}
Solution 2
You could also use TByteArrayList from the GNU Trove library.
Solution 3
You have a Byte
class provided by the JRE.
This class is the corresponding class for the byte
primitive type.
See here for primitive types.
You can do this :
List<Byte> myList = new ArrayList<Byte>();
byte b = 127;
myList.add(b);
b = 0; // resetting
b = myList.get(0); // => b = 127 again
As Michael pointed in the comments :
List<Byte> myList = new ArrayList<Byte>();
Byte b = null;
myList.add(b);
byte primitiveByte = myList.get(0);
results in :
Exception in thread "main" java.lang.NullPointerException
at TestPrimitive.main(TestPrimitive.java:12)
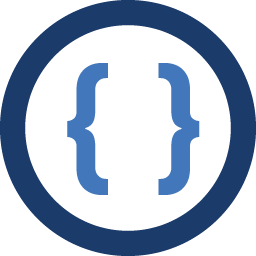
Author by
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I want to make a growable array of bytes. I.e a list. In c# would usally do the following syntax
List<byte> mylist = new List<byte>();
where as in java this syntax does not work and I have googled around and found the below code
List myList = new ArrayList();
but that is not what I want. Any idea's where I am going wrong?