Java - get the newest file in a directory?
109,130
Solution 1
The following code returns the last modified file or folder:
public static File getLastModified(String directoryFilePath)
{
File directory = new File(directoryFilePath);
File[] files = directory.listFiles(File::isFile);
long lastModifiedTime = Long.MIN_VALUE;
File chosenFile = null;
if (files != null)
{
for (File file : files)
{
if (file.lastModified() > lastModifiedTime)
{
chosenFile = file;
lastModifiedTime = file.lastModified();
}
}
}
return chosenFile;
}
Note that it required Java 8
or newer due to the lambda expression.
Solution 2
In Java 8:
Path dir = Paths.get("./path/somewhere"); // specify your directory
Optional<Path> lastFilePath = Files.list(dir) // here we get the stream with full directory listing
.filter(f -> !Files.isDirectory(f)) // exclude subdirectories from listing
.max(Comparator.comparingLong(f -> f.toFile().lastModified())); // finally get the last file using simple comparator by lastModified field
if ( lastFilePath.isPresent() ) // your folder may be empty
{
// do your code here, lastFilePath contains all you need
}
Solution 3
This works perfectly fine for me:
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.comparator.LastModifiedFileComparator;
import org.apache.commons.io.filefilter.WildcardFileFilter;
...
/* Get the newest file for a specific extension */
public File getTheNewestFile(String filePath, String ext) {
File theNewestFile = null;
File dir = new File(filePath);
FileFilter fileFilter = new WildcardFileFilter("*." + ext);
File[] files = dir.listFiles(fileFilter);
if (files.length > 0) {
/** The newest file comes first **/
Arrays.sort(files, LastModifiedFileComparator.LASTMODIFIED_REVERSE);
theNewestFile = files[0];
}
return theNewestFile;
}
Solution 4
private File getLatestFilefromDir(String dirPath){
File dir = new File(dirPath);
File[] files = dir.listFiles();
if (files == null || files.length == 0) {
return null;
}
File lastModifiedFile = files[0];
for (int i = 1; i < files.length; i++) {
if (lastModifiedFile.lastModified() < files[i].lastModified()) {
lastModifiedFile = files[i];
}
}
return lastModifiedFile;
}
Solution 5
Something like:
import java.io.File;
import java.util.Arrays;
import java.util.Comparator;
public class Newest {
public static void main(String[] args) {
File dir = new File("C:\\your\\dir");
File [] files = dir.listFiles();
Arrays.sort(files, new Comparator(){
public int compare(Object o1, Object o2) {
return compare( (File)o1, (File)o2);
}
private int compare( File f1, File f2){
long result = f2.lastModified() - f1.lastModified();
if( result > 0 ){
return 1;
} else if( result < 0 ){
return -1;
} else {
return 0;
}
}
});
System.out.println( Arrays.asList(files ));
}
}
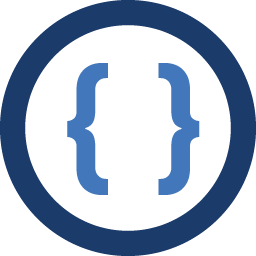
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Does anybody have a snippet of Java that can return the newest file in a directory (or knowledge of a library that simplifies this sort of thing)?
-
Chris Conway over 15 yearsIt's kind of wonky to force a ClassCastException in the non-File case, instead of, say, asserting instanceof.
-
OscarRyz over 15 yearsYou won't get a non-File from the array returned by File.listFiles();
-
Zach Scrivena over 15 yearsRemember to check that listFiles() doesn't return null.
-
andrej over 10 yearsc'mon, you don't have to SORT array to get minimum value!
-
andrej over 10 yearsc'mon, you don't have to SORT array to get minimum value!
-
mes over 9 yearsMaybe perfect, but no fast
-
Cthulhu almost 9 yearsPlease don't just dump your code. Explain your train of thought so we can better understand your answer. Thanks.
-
Kiet Tran over 8 yearsWhat happens if the difference between the two last-modified timestamps is larger than Integer.MAX_VALUE?
-
nilsmagnus over 8 yearsThere is a typo in your example: isPresented() should be isPresent()
-
Admin almost 8 yearsWhy don't you use new Comparator<File>()? This avoids the cast and the extra function.
-
Dileep over 7 yearsWhat if we are not updating the directory it will give the same result every time.?
-
Prasanth V almost 7 yearsguys you can use this code for get last modified file.
-
RaviSam over 3 yearsis it possible without loading full files list? In cases when directory is too large.