Java:how to pass value from class/bean to servlet
You don't pass something into a servlet. You just let the servlet access something.
You should get rid of that main()
method and move the database interaction code into a DAO class. I'd also give the model class (with timezone and location) a more sensitive name starting with an uppercase. So all with all, you should update the code so that it look something like the following:
Model class, the Area
(name it whatever you want, as long as it makes sense), it should just represent a single entity:
public class Area {
private String location;
private String timezone;
public String getLocation() { return location; }
public String getTimezone() { return timezone; }
public void setLocation(String location) { this.location = location; }
public void setTimezone(String timezone) { this.timezone = timezone; }
}
Basic connection manager class, the Database
, here you load the driver just once and provide a getter for the connection:
public class Database {
private String url;
private String username;
private String password;
public Database(String driver, String url, String username, String password) {
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
throw new RuntimeException("Driver class is missing in classpath", e);
}
this.url = url;
this.username = username;
this.password = password;
}
public Connection getConnection() {
return DriverManager.getConnection(url, username, password);
}
}
DAO class, the AreaDAO
, here you put all DB interaction methods:
public class AreaDAO {
private Database database;
public AreaDAO(Database database) {
this.database = database;
}
public List<Area> list() throws SQLException {
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
List<Area> areas = new ArrayList<Area>();
try {
connection = database.getConnection();
statement = connection.prepareStatement("SELECT location, timezone FROM userclient");
resultSet = statement.executeQuery();
while (resultSet.next()) {
Area area = new Area();
area.setLocation(resultSet.getString("location"));
area.setTimezone(resultSet.getString("timezone"));
areas.add(area);
}
} finally {
if (resultSet != null) try { resultSet.close(); } catch (SQLException logOrIgnore) {}
if (statement != null) try { statement.close(); } catch (SQLException logOrIgnore) {}
if (connection != null) try { connection.close(); } catch (SQLException logOrIgnore) {}
}
return areas;
}
}
Finally, in the servlet initialize the DAO once and obtain the list in the HTTP method:
public class AreaServlet extends HttpServlet {
private AreaDAO areaDAO;
public void init() throws ServletException {
String driver = "com.mysql.jdbc.Driver";
String url = "jdbc:mysql://localhost:3306/dbname";
String username = "user";
String password = "pass";
Database database = new Database(driver, url, username, password);
this.areaDAO = new AreaDAO(database);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
List<Area> areas = areaDAO.list();
request.setAttribute("areas", areas);
request.getRequestDispatcher("/WEB-INF/areas.jsp").forward(request, response);
} catch (SQLException e) {
throw new ServletException("Cannot retrieve areas", e);
}
}
}
Map this servlet on an url-pattern
of /areas
in web.xml
so that you can invoke it by http://example.com/contextname/areas
The /WEB-INF/areas.jsp
can look like something this, assuming that you want to display the areas in a table:
<table>
<c:forEach items="${areas}" var="area">
<tr>
<td>${area.location}</td>
<td>${area.timezone}</td>
</tr>
</c:forEach>
</table>
See also:
- Beginning and intermediate JSP/Servlet tutorials
- Advanced JSP/Servlet tutorials
- DAO tutorial (contains more advanced/flexible DAO examples)
- Hidden features of JSP/Servlet
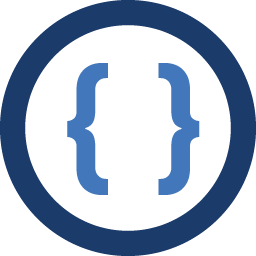
Admin
Updated on June 23, 2022Comments
-
Admin almost 2 years
i am new to java, i'm having problem passing value from a class/bean (which are stored in arraylist) to servlet. any idea how can i achieve that? below is my code.
package myarraylist; public class fypjdbClass { String timezone; String location; public String getTimezone() { return timezone; } public void setTimezone(String timezone) { this.timezone = timezone; } public String getLocation() { return location; } public void setLocation(String location) { this.location = location; } public fypjdbClass() { super(); ArrayList<fypjdbClass> fypjdbList = new ArrayList<fypjdbClass>(); this.timezone = timezone; this.location = location; } public static void main(String[] args) { //Establish connection to MySQL database String connectionURL = "jdbc:mysql://localhost/fypjdb"; Connection connection=null; ResultSet rs; try { // Load the database driver Class.forName("com.mysql.jdbc.Driver"); // Get a Connection to the database connection = DriverManager.getConnection(connectionURL, "root", ""); ArrayList<fypjdbClass> fypjdbList = new ArrayList<fypjdbClass>(); //Select the data from the database String sql = "SELECT location,timezone FROM userclient"; Statement s = connection.createStatement(); //Create a PreparedStatement PreparedStatement stm = connection.prepareStatement(sql); rs = stm.executeQuery(); //rs = s.getResultSet(); while(rs.next()){ fypjdbClass f = new fypjdbClass(); f.setTimezone(rs.getString("timezone")); f.setLocation(rs.getString("location")); fypjdbList.add( f); } for (int j = 0; j < fypjdbList.size(); j++) { System.out.println(fypjdbList.get(j)); } //To display the number of record in arraylist System.out.println("ArrayList contains " + fypjdbList.size() + " key value pair."); rs.close (); s.close (); }catch(Exception e){ System.out.println("Exception is ;"+e); } } }
And this is the Servlet
package myarraylist; public class arraylistforfypjServlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public arraylistforfypjServlet() { super(); } public static ArrayList<fypjdbClass> fypjdbList = new ArrayList<fypjdbClass>(); /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //processRequest(request, response); RequestDispatcher rd = request.getRequestDispatcher("/DataPage.jsp"); //You could give a relative URL, I'm just using absolute for a clear example. ArrayList<fypjdbClass> fypjdbList = new ArrayList<fypjdbClass>();// You can use any type of object you like here, Strings, Custom objects, in fact any object. request.setAttribute("fypjdbList", fypjdbList); rd.forward(request, response); } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //processRequest(request, response); doGet(request,response); } }
i dont know if my code is right, pls advise me. thanks lot ^^