Java - Recursion sum of number and how it work
17,179
Solution 1
Instead of setting the sum to 0
you can -
Do this:
public int sumUp(int n){
if (n==1)
return 1;
else
return sumUp(n-1)+n;
}
Solution 2
The problem is you set the sum always 0.
public static void main(String[] args) {
System.out.println(num(5, 0));
}
public static int num(int n, int sum) {
if (n == 0) {
return sum;
}
sum += n;
return num(n - 1, sum);
}
Solution 3
public static int withRecursion(List<Integer> list) {
int size = list.size();
int a=0;
if(list.isEmpty() == true) {
return 0;
}else {
a = a + list.get(0) + withRecursion(list.subList(1, size));
return a;
}
}
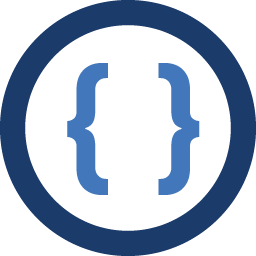
Author by
Admin
Updated on June 06, 2022Comments
-
Admin almost 2 years
I am trying to write a recursive function that when I call with number 5 for example then the function will calculate the sum of all digits of five. 1 + 2 + 3 + 4 + 5 = 15
The current code always returns 0, how can the amount each time the n?
public class t { public static void main(String[] args) { System.out.println(num(5)); } public static int num(int n) { int sum = 0; sum += n; if (n == 0) return sum; return num(n - 1); } }
thank you.
-
zapl almost 8 years@KevinWallis and that's not even all you can do.
return n <= 1 ? n : num(n -1) + n;
does it in 1 line. -
Tilak Madichetti almost 8 yearshe just seems to be .. learning java ; why do you wanna confuse him with that anyways, i'll add it to my answer..