javascript - time counter since start date and time
Solution 1
From your question I understand you store the date and time on start? So then you can use PHP to echo this information in a starting Date object and let a setInterval-function do the current timegetting and calculation of the time working.
See working example here: http://jsfiddle.net/c0rxkhyz/1/
This is the code:
var startDateTime = new Date(2014,0,1,23,59,59,0); // YYYY (M-1) D H m s ms (start time and date from DB)
var startStamp = startDateTime.getTime();
var newDate = new Date();
var newStamp = newDate.getTime();
var timer; // for storing the interval (to stop or pause later if needed)
function updateClock() {
newDate = new Date();
newStamp = newDate.getTime();
var diff = Math.round((newStamp-startStamp)/1000);
var d = Math.floor(diff/(24*60*60)); /* though I hope she won't be working for consecutive days :) */
diff = diff-(d*24*60*60);
var h = Math.floor(diff/(60*60));
diff = diff-(h*60*60);
var m = Math.floor(diff/(60));
diff = diff-(m*60);
var s = diff;
document.getElementById("time-elapsed").innerHTML = d+" day(s), "+h+" hour(s), "+m+" minute(s), "+s+" second(s) working";
}
timer = setInterval(updateClock, 1000);
<div id="time-elapsed"></div>
Attention! The month number in the new Date()
declaration is minus one (so January is 0, Feb 1, etc)!
Solution 2
I would use momentJS fromNow function. You can get the time started as variable on page load then call fromNow on that and current time to get time between the two every time the clock is clicked:
var StartedWorkDateTime = GetStartedTime();
moment(StartedWorkDateTime).fromNow(true);
Non momentJS:
var date1 = new Date("7/11/2010 15:00");
var date2 = new Date("7/11/2010 18:00");
var timeDiff = Math.abs(date2.getTime() - date1.getTime());
var diffHours = Math.ceil(timeDiff / (1000 * 3600));
alert(diffHours);
Solution 3
Get the difference between two dates by subtracting them:
var duration = end - start;
This will give you the number of milliseconds between the dates. You can use the milliseconds to figure out hours, minutes, and seconds. Then it's just a matter of string manipulation and writing the value to the page. To update the timer once per second, use setInterval()
:
setInterval(writeDuration, 1000);
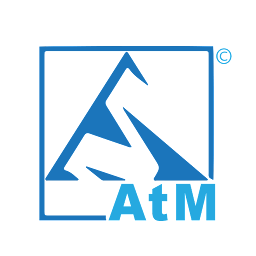
Richard Jones
Updated on June 28, 2022Comments
-
Richard Jones almost 2 years
I have a new business, where I just hired someone to work for me, I am trying to make it easy for her to track her time, so I created a clock in button, that creates a record in a database, I have it pop up a small window, that she can click to clockout when she is done working.
I want it to show her on that popup window a counter that will show how long she has been working, so I want to create a javascript or jQuery that will start at a certain time and count from there. She is on the East Coast, our company is in the Central Timezone, so 1 hour behind her.
How can I get a javascript to start from a certain time and keep updating the timer, so she can see something like this:
[You've been working for: 01:01:01 HH::MM::SS] - and it is actively updating, climbing up.
All the timers I've found are not about time itself, but about starting at a time and counting down, or starting at 0 and counting up.
Is there a way to tell it a start time, so that way if she reloads the page, it does not start from 0, but will start at the time she clocked in, then add the time since and start from there?
I know it can be done, but I'm more of a Perl guy than a Javascript guy. I'm doing this on Wordpress, so I could use PHP and just tell her to refresh the page to see the current amount of time and then have it on page load show the current amount of time, but I think having a counter would be better and make it easier for her.
is there some code already done that I could modify myself to make it work? I cannot find any, anywhere. I'm willing to do all the work, I'm not asking for someone to do it for me.
I found this example someone did:
function get_uptime() { var t1 = new Date() var t2 = new Date() var dif = t1.getTime() - t2.getTime() seconds = dif / 1000; Seconds_Between_Dates = Math.abs(seconds); document.getElementById("seconds").innerHTML = Seconds_Between_Dates; setTimeout(get_uptime, 1000); } get_uptime();
That is sort of it, but I don't now how to put the first time in t1, what format do I put it in? I can have PHP put it in any format, but not sure the one it needs. Plus this appears to only put the seconds, not hours, minutes and seconds.
Is there away to do that?
Thanks, Richard