Jersey, Tomcat: The requested resource is not available error
You are deploying a compiled code to Tomcat, so you won't be able to access the *.java resources.
Annotation @Path("/hello")
indicates the path at which resource is available.
It is set to: base URL + /your_path
. The base URL
is based on your application name, the servlet and the URL pattern from the web.xml
:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="jersey" version="2.5">
<servlet>
<servlet-name>jersey</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>jersey</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>jersey</servlet-name>
<url-pattern>/*</url-pattern>
</servlet-mapping>
</web-app>
Also replace @Produces
annotation to @Consumes
:
package jersey;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class Hello {
// This method is called if TEXT_PLAIN is request
@GET
@Consumes(MediaType.TEXT_PLAIN)
public String sayPlainTextHello() {
return "Hello Jersey";
}
// This method is called if XML is request
@GET
@Consumes(MediaType.TEXT_XML)
public String sayXMLHello() {
return "<?xml version=\"1.0\"?>" + "<hello> Hello Jersey" + "</hello>";
}
@GET
@Consumes(MediaType.TEXT_HTML)
public String sayHtmlHello() {
return "<html> " + "<title>" + "Hello Jersey" + "</title>"
+ "<body><h1>" + "Hello Jersey" + "</body></h1>" + "</html> ";
}
}
Try: http://localhost:8080/jersey/hello
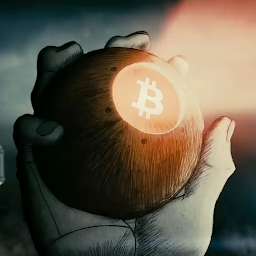
Comments
-
Jake Schuurmans about 2 years
I have been working towards getting a RESTful service set up using Jersey and Tomcat in RAD 8.5. I have looked at tons of stackoverflow questions related to my error with none of them working. There are no errors in my console.
When I just type: http://localhost:8080/, I get the Apache homepage, so the server is working, but http://localhost:8080/jersey/rest/hello or http://localhost:8080/jersey/WEB-INF/classes/jersey/Hello.java does not work.
Here is the error: (with my library of jars on the side)
Here is my
Hello.java
package jersey; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; @Path("/hello") public class Hello { // This method is called if TEXT_PLAIN is request @GET @Produces(MediaType.TEXT_PLAIN) public String sayPlainTextHello() { return "Hello Jersey"; } // This method is called if XML is request @GET @Produces(MediaType.TEXT_XML) public String sayXMLHello() { return "<?xml version=\"1.0\"?>" + "<hello> Hello Jersey" + "</hello>"; } @GET @Produces(MediaType.TEXT_HTML) public String sayHtmlHello() { return "<html> " + "<title>" + "Hello Jersey" + "</title>" + "<body><h1>" + "Hello Jersey" + "</body></h1>" + "</html> "; } }
And my web.xml
<servlet> <servlet-name>Jersey REST Service</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>com.example</param-value> </init-param> </servlet>
Versions:
- Tomcat: 7.0.663
- RAD: 8.5
- Jersey: 2.19
Thanks,
In Response to Maciej This worked! I needed to add
<servlet-mapping>
with url pattern of/*
. Then use http://localhost:8080/jersey/hello, I got a response from the server!<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="jersey" version="2.5"> <servlet> <servlet-name>jersey</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>jersey</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>jersey</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping> </web-app>
-
Jake Schuurmans almost 9 yearsSame thing happens,
The requested resource is not available
-
Maciej Lach almost 9 yearsTry to configure the servlet mapping to match:
jersey/*
-
Jake Schuurmans almost 9 yearsIm going to answer in my question
-
Maciej Lach almost 9 years
servlet-mapping
tag should defined on the same level asservlet
-
Jake Schuurmans almost 9 yearsNow my server will not start. I get a couple of
Failed to start component [StandardEngine[Catalina].StandardHost[localhost].StandardContext[/jersey]]
errors -
Maciej Lach almost 9 yearsSorry, the servlet mapping should match
*
(or remove it). You need to verifybase URL
, it should be same as name of the war archive or folder in the Tomcatwebapps
. -
Jake Schuurmans almost 9 yearsIn the Tomcat server? or where i installed Tomcat? because i cant find a war file anywhere =/.
-
Maciej Lach almost 9 yearsIf you run from Eclipse check this post for details on location of the deployment folder: stackoverflow.com/questions/3515089/…
-
Maciej Lach almost 9 yearsOne more thing: there is an error in the package configuration where Jersey scans for packages:
<param-value>com.example</param-value>
should be set to<param-value>jersey</param-value>
. I'll update the answer in few minutes. -
Jake Schuurmans almost 9 yearsI found a war file, it is sample.war. how do i reference it in <url-pattern>? like so
<url-pattern>docs\appdev\</url-pattern>
? -
Jake Schuurmans almost 9 yearsjust did, still nothing, in order to run the server i had to comment out your servlet-mapping addition, it stopped my server from starting.
-
Maciej Lach almost 9 yearsI've updated the post: servlet mapping has to be set to
/*
. Also there was a slight change in Hello.java regarding annotations.