Laravel display validation error
11,527
Solution 1
Ok, I found mistake. I didn't have another language to upload. In file app/config.php replace 'locale' => 'pl' to 'en'
Solution 2
you need to add validation in controller method like this..
$request->validate([
'name' => 'required'
]);
then you can show your validation error in view :
@if ($errors->has('name'))
<li>{{ $errors->first('name') }}</li>
@endif
and this should also work ...
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
Solution 3
Here is a good example of handling errors in laravel
message.blade.php
@if($errors->any())
<div class="alert alert-danger">
<p><strong>Opps Something went wrong</strong></p>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
@if(session('success'))
<div class="alert alert-success">{{session('success')}}</div>
@endif
@if(session('error'))
<div class="alert alert-danger">{{session('error')}}</div>
@endif
In your controller update method for instance,
public function update(Request $request, $id)
{
$this->validate($request,[
'title'=>'required',
'body'=>'required'
]);
//the above validation is important to get the errors caught
$post= Post::find($id);
$post->title = $request->input('title');
$post->body = $request->input('body');
$post->save();
return redirect('/posts')->with('success','Updated successfully');
}
if you have a layout file as layout.blade.php NOTE: having the error display in the layout file is advantageous to use the message for all purpose.
...
<div class="container">
@include('message')
@yield('content')
</div>
...
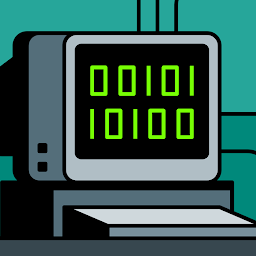
Author by
grantDEV
Updated on June 05, 2022Comments
-
grantDEV almost 2 years
I try use this code:
@foreach($errors->all() as $error) <li>{!! $error !!}</li> @endforeach
I would like the error to be displayed correctly. exemple The name is required.
In debbuger I can see. How to replace validation.required upon "The name is required"
-
Maky almost 6 yearsHave you returned the errors in the controller with an validator?
-
grantDEV almost 6 yearsI make new request and I wrote in controller -> return redirect('route');
-
Maky almost 6 yearssee the answers below with $request->validate method
-
Ron van der Heijden almost 6 yearsIf you return after the submission, ofcourse Laravel loses the data. What did you expect? If you realy want to redirect, do it with flashed session data.
-
grantDEV almost 6 yearsOk, I know. An error from the validator is displayed to me in a view only in a strange form such as "validation.reqiured". See screen above.
-
-
Ron van der Heijden almost 6 yearsThis is not how you should validate form submission.
-
8bitIcon almost 5 years@Ankit Why do we need to chain
all()
? If$errors
is an array we can simply loop using foreach. -
Ankit24007 almost 5 years@SujeetAgrahari
$errors
is not an array here,$errors
is an object which consist all the validation errors, you can check, get and display the errors through the methods which errors class provide.