Matlab axes scaling
Solution 1
If you want to see your new plotted data replace the old plotted data, but maintain the same axes limits, you can update the x and y values of the plotted data using the SET command within your loop. Here's a simple example:
hAxes = axes; %# Create a set of axes
hData = plot(hAxes,nan,nan,'*'); %# Initialize a plot object (NaN values will
%# keep it from being displayed for now)
axis(hAxes,[0 2 0 4]); %# Fix your axes limits, with x going from 0
%# to 2 and y going from 0 to 4
for iLoop = 1:200 %# Loop 100 times
set(hData,'XData',2*rand,... %# Set the XData and YData of your plot object
'YData',4*rand); %# to random values in the axes range
drawnow %# Force the graphics to update
end
When you run the above, you will see an asterisk jump around in the axes for a couple of seconds, but the axes limits will stay fixed. You don't have to use the HOLD command because you are just updating an existing plot object, not adding a new one. Even if the new data extends beyond the axes limits, the limits will not change.
Solution 2
You have to set the axes limits; ideally you do that before starting the loop.
This won't work
x=1:10;y=ones(size(x)); %# create some data
figure,hold on,ah=gca; %# make figure, set hold state to on
for i=1:5,
%# use plot with axis handle
%# so that it always plots into the right figure
plot(ah,x+i,y*i);
end
This will work
x=1:10;y=ones(size(x)); %# create some data
figure,hold on,ah=gca; %# make figure, set hold state to on
xlim([0,10]),ylim([0,6]) %# set the limits before you start plotting
for i=1:5,
%# use plot with axis handle
%# so that it always plots into the right figure
plot(ah,x+i,y*i);
end
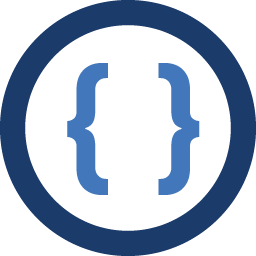
Admin
Updated on July 24, 2020Comments
-
Admin almost 4 years
how exactly do you get fixed scaling of axes in Matlab plot when plotting inside a loop? My aim is to see how data is evolving inside the loop. I tried using
axis manual
andaxis(...)
with no luck. Any suggestions?I know
hold on
does the trick, but I don't want to see the old data. -
Amro over 13 years+1 I also have a couple of suggestions: 1) to avoid flickering, you should enable double buffering
set(gcf,'DoubleBuffer','on')
. 2) if you want to increase the speed of drawing and get a smoother animation, set theEraseMode
property to something other than 'normal' (I would usexor
in this case). Of course you'll have to use low-level functions like line,patch,text,etc.. Check out this guide for more details: mathworks.com/support/tech-notes/1200/1204.html#Section%2023