Method not found in class
Solution 1
PhpStorm cannot figure out what type your $this->cxn
field is. You can help by providing typehint via simple PHPDoc comment:
/** @var Database */
private $cxn; //database object
Solution 2
also this hint is helping PHPstorm to recognize the methods correctly
if you have code like this:
foreach( $myEntity as $entity ) {
$entity->myValidMethod();
}
just add this hint:
/* @var $entity \My\Name\Space\Path\MyEntity */
foreach( $myEntity as $entity ) {
$entity->myValidMethod();
}
thanks to http://mossco.co.uk/symfony-2/how-to-fix-method-not-found-in-class-orange-warning-in-phpstorm/
Solution 3
I don't think there are visibility issues in the code. The PHP manual states:
Class methods may be defined as public, private, or protected. Methods declared without any explicit visibility keyword are defined as public.
I think you might have two Database classes. Similar issue was described in this topic: best way to call function from an extended class
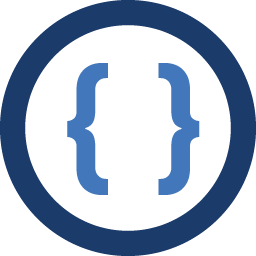
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I'm working on a login function but got an error that I can't figure out.
This is my Model Login class:
class Login { private $username; private $password; private $cxn; //database object function __construct($username,$password) { //set data $this->setData($username, $password); //connect DB $this->connectToDB(); // get Data } function setData($username, $password) { $this->username = $username; $this->password = $password; } private function connectToDB() { include 'Database.php'; $connect = '../include/connect.php'; $this->cxn = new database($connect); } function getData() { $query = "SELECT * FROM anvandare WHERE anvandarnamn = '$this->username' AND losenord ='$this->'password'"; $sql = mysql_query($query); if(mysql_num_rows($sql)>0) { return true; } else { throw new Exception("Användarnamnet eller Lösenordet är fel. Försök igen."); } } function close() { $this->cxn->close(); //Here is the error it says "Method 'close' not found in class } }
The last function gives error "Method 'close' not found in class", "Referenced method is not found in subject class."
Here is my Database class:
class Database { private $host; private $user; private $password; private $database; function __construct($filename) { if(is_file($filename)) include $filename; else throw new exception("Error!"); $this->host=$host; $this->user=$user; $this->password=$password; $this->database=$database; $this->connect(); } private function connect() { //Connect to the server if(!mysql_connect($this->host, $this->user, $this->password)) throw new exception("Error, not connected to the server."); //Select the database if(!mysql_select_db($this->database)) throw new exception("Error, no database selected"); } function close() { mysql_close(); } }
The pathways are correct so it's not it. What could be the error?