Multiple providers in a single vagrant file?
Solution 1
You can use a multi-vm environment, where every VM can be provisioned with a different provider and you can choose on commandline which one you want to vagrant up <machine>
.
Solution 2
add box for each provider
> vagrant box add precise64 http://file.vagrantup.com/precise64.box
> vagrant box add precise64 http://file.vagrantup.com/precise64_vmware_fusion.box
and your Vagrantfile should look like
Vagrant.configure(2) do |config|
config.vm.box="precise64"
config.vm.provider "virtualbox" do |v|
v.customize ["modifyvm", :id, "--memory", "2048"]
end
config.vm.provider "vmware_fusion" do |v|
v.vmx["memsize"] = "2048"
end
end
then create on each provider using following commands
> vagrant up --provider=virtualbox
> vagrant up --provider=vmware_fusion
Solution 3
You can choose which provider you want to run by --provider
parameter.
Here is ruby code in Vagrantfile
which can run different VM depending which provider you have chosen:
require 'getoptlong'
# Parse CLI arguments.
opts = GetoptLong.new(
[ '--provider', GetoptLong::OPTIONAL_ARGUMENT ],
)
provider='virtualbox'
begin
opts.each do |opt, arg|
case opt
when '--provider'
provider=arg
end # case
end # each
rescue
end
# Vagrantfile API/syntax version.
Vagrant.configure(2) do |config|
config.vm.hostname = "vagrant"
config.vm.define "default-#{provider}"
config.vm.provider "virtualbox" do |vbox, override|
vbox.customize ['modifyvm', :id, '--natdnshostresolver1', 'on']
vbox.name = "test.local"
override.vm.box = "ubuntu/wily64"
override.vm.network "private_network", ip: "192.168.22.22"
end
config.vm.provider :aws do |aws, override|
aws.ami = "ami-7747d01e"
aws.instance_type = "m3.medium"
override.vm.box = "dummy"
override.vm.box_url = "https://github.com/mitchellh/vagrant-aws/raw/master/dummy.box"
override.ssh.username = "ubuntu"
end
end
So by the default provider is virtualbox, but you can specify from the command line, like:
vagrant up --provider=aws
Solution 4
To run VM locally you can run:
vagrant up --provider=virtualbox
and if you'd like to run VM using different provider then you can use:
vagrant up --provider=aws
However, remember that you have to install appropriate provider plugin before you will use it.
Solution 5
Please check this gist posted by @tknerr which works with all providers such as virtualbox, AWS and managed in combination with the vagrant-omnibus plugin. Here is the code from Vagrantfile
:
#
# Vagrantfile for testing
#
Vagrant::configure("2") do |config|
# the Chef version to use
config.omnibus.chef_version = "11.4.4"
def configure_vbox_provider(config, name, ip, memory = 384)
config.vm.provider :virtualbox do |vbox, override|
# override box url
override.vm.box = "opscode_ubuntu-13.04_provisionerless"
override.vm.box_url = "https://opscode-vm.s3.amazonaws.com/vagrant/opscode_ubuntu-13.04_provisionerless.box"
# configure host-only network
override.vm.hostname = "#{name}.local"
override.vm.network :private_network, ip: ip
# enable cachier for local vbox vms
override.cache.auto_detect = true
# virtualbox specific configuration
vbox.customize ["modifyvm", :id,
"--memory", memory,
"--name", name
]
end
end
def configure_aws_provider(config)
config.vm.provider :aws do |aws, override|
# use dummy box
override.vm.box = "aws_dummy_box"
override.vm.box_url = "https://github.com/mitchellh/vagrant-aws/raw/master/dummy.box"
# override ssh user and private key
override.ssh.username = "ubuntu"
override.ssh.private_key_path = "#{ENV['HOME']}/.ssh/mccloud_rsa"
# aws specific settings
aws.access_key_id = "XXXX"
aws.secret_access_key = "XXXXX"
aws.ami = "ami-524e4726"
aws.region = "eu-west-1"
aws.availability_zone = "eu-west-1c"
aws.instance_type = "m1.small"
aws.security_groups = [ "mccloud", "http" ]
aws.keypair_name = "mccloud-key-tlc"
end
end
def configure_managed_provider(config, server)
config.vm.provider :managed do |managed, override|
# use dummy box
override.vm.box = "managed_dummy_box"
override.vm.box_url = "https://github.com/tknerr/vagrant-managed-servers/raw/master/dummy.box"
# link with this server
managed.server = server
end
end
#
# define a separate VMs for the 3 providers (vbox, aws, managed)
# because with Vagrant 1.2.2 you can run a VM with only one provider at once
#
[:aws, :vbox, :esx].each do |provider|
#
# Sample VM per provider
#
config.vm.define :"sample-app-#{provider}" do | sample_app_config |
case provider
when :vbox
configure_vbox_provider(sample_app_config, "sample-app", "33.33.40.10")
when :aws
configure_aws_provider(sample_app_config)
when :esx
configure_managed_provider(sample_app_config, "33.33.77.10")
end
sample_app_config.vm.provision :chef_solo do |chef|
chef.cookbooks_path = [ './cookbooks/sample-app-0.1.0' ]
chef.add_recipe "sample-app"
chef.json = {
:sample_app => {
:words_of_wisdom => "Vagrant on #{provider} Rocks!"
}
}
end
end
end
end
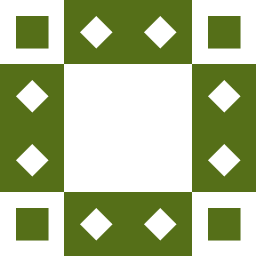
jbrown
Updated on June 28, 2022Comments
-
jbrown 4 months
I've got a vagrant file that builds a local VM. I want to add the EC2 provider and have the option of either provisioning a local VM or one on EC2.
Can I create configs for multiple providers in the same Vagrantfile and somehow choose which to run when I do
vagrant up
? -
Peeja almost 9 yearsFor future readers: I've edited the question to call them "providers" rather than "provisioners".
-
rravuri almost 7 yearsthe question was about multiple 'providers' not 'provisioners'
-
mehmet over 6 yearsJust note you cannot have multiple providers running at the same time. In that case you must use multi machine environment.
-
hekevintran about 5 yearsThe question was originally asked about provisioners and then edited to ask about providers.