Multiple statements found while compiling a single statement
You have a few indentation problems. In python indentation is very important, because the interpreter uses indentation levels to decide how to group statements. for example:
if (False):
print("Hello")
print("World")
The statement(s) grouped with the if (False) statement should never be ran because if (False) should never be true. But the example I gave you will still output "World". This is because the interpreter doesn't see the second print statement as being a part of the if statement. Now if I were to take the exact same code and indent the second print statement like so:
if (False):
print("Hello")
print("World")
The interpreter will see that both print statements are one indentation level deeper than the if statement and nothing will be outputted because if (False) is always false.
The same indentation applies to function definitions. For example:
def foo():
if(True):
print("Hello, World")
Because the if statement is indented one level deeper that the definition of foo it is grouped with the foo function. So when you call the foo function it will output "Hello, World".
Now for variables. In your code you have the variable indented in one level. Which would be fine if it were a part of a function definition, if statement, for loop, etc. But since it isn't, it creates problems. Take the following for example:
a="Hello, World"
if(True):
print(a)
Will give you a syntax or indentation error, While:
a="Hello, World"
if(True):
print(a)
Will print "Hello, World".
Now to focus on your code:
SUFFIXES = {1000: ['KB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB'],
1024: ['KiB', 'MiB', 'GiB', 'TiB', 'PiB', 'EiB', 'ZiB', 'YiB']}
Needs to be un-indented one level to become:
SUFFIXES = {1000: ['KB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB'],
1024: ['KiB', 'MiB', 'GiB', 'TiB', 'PiB', 'EiB', 'ZiB', 'YiB']}
Also:
def approximate_size(size, a_kilobyte_is_1024_bytes=True):
Needs to be un-indented one level to become:
def approximate_size(size, a_kilobyte_is_1024_bytes=True):
And:
if __name__ == '__main__':
print(approximate_size(1000000000000, False))
print(approximate_size(1000000000000))
Needs to be:
if __name__ == '__main__':
print(approximate_size(1000000000000, False))
print(approximate_size(1000000000000))
Throw all of this together and you get:
SUFFIXES = {1000: ['KB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB'],
1024: ['KiB', 'MiB', 'GiB', 'TiB', 'PiB', 'EiB', 'ZiB', 'YiB']}
def approximate_size(size, a_kilobyte_is_1024_bytes=True):
'''Convert a file size to human-readable form.
Keyword arguments:
size -- file size in bytes
a_kilobyte_is_1024_bytes -- if True (default), use multiples of 1024
if False, use multiples of 1000
Returns: string
'''
if size < 0:
raise ValueError('number must be non-negative')
multiple = 1024 if a_kilobyte_is_1024_bytes else 1000
for suffix in SUFFIXES[multiple]:
size /= multiple
if size < multiple:
return '{0:.1f} {1}'.format(size, suffix)
raise ValueError('number too large')
if __name__ == '__main__':
print(approximate_size(1000000000000, False))
print(approximate_size(1000000000000))
I hope this helps!
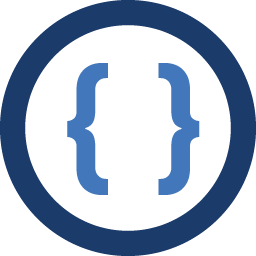
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am brand new to programming. I am using the Dive into Python book and am trying to run the first example, humansize.py. I have copied and pasted the code into Idle, the Python shell and keep coming up with the same syntax error: "multiple statements found while compiling a single statement."
I am downloading the code into BBEdit and then copying and pasting it into Idle. I have looked online and people said it could be a tab versus space issue. But I've went through the code and it looks identical to the book, I've even deleted and reinserted 4 spaces in all the lines of code and I'm still getting the error.
It's frustrating because I am sure that it's a simple issue but I've done everything that I know of, (in terms of trying to research the problem) to get it to work. If it is a space versus tabs issue, do any of you know where I can go and learn how to do the process of copying and entering code into Idle properly? I am a TRUE beginner.
I'd appreciate any assistance from the community. Thank you!
I am running a Mac OSX - V.10.7.5. I am using the latest version of the Dive into Python book and Python 3.3.
The code is below:
>>> '''Convert file sizes to human-readable form. Available functions: approximate_size(size, a_kilobyte_is_1024_bytes) takes a file size and returns a human-readable string Examples: >>> approximate_size(1024) '1.0 KiB' >>> approximate_size(1000, False) '1.0 KB' ''' SUFFIXES = {1000: ['KB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB'], 1024: ['KiB', 'MiB', 'GiB', 'TiB', 'PiB', 'EiB', 'ZiB', 'YiB']} def approximate_size(size, a_kilobyte_is_1024_bytes=True): '''Convert a file size to human-readable form. Keyword arguments: size -- file size in bytes a_kilobyte_is_1024_bytes -- if True (default), use multiples of 1024 if False, use multiples of 1000 Returns: string ''' if size < 0: raise ValueError('number must be non-negative') multiple = 1024 if a_kilobyte_is_1024_bytes else 1000 for suffix in SUFFIXES[multiple]: size /= multiple if size < multiple: return '{0:.1f} {1}'.format(size, suffix) raise ValueError('number too large') if __name__ == '__main__': print(approximate_size(1000000000000, False)) print(approximate_size(1000000000000)) **SyntaxError: multiple statements found while compiling a single statement** >>>
-
Admin over 10 yearsThank you, jYaeger! It certainly does help a lot! I've finally got it to run and am learning more about how tabs affects the runing of programs. Your explanation was thorough and invaluable. I appreciate your help!