multiple tasks using python asyncio
12,449
I was looking for examples and I found the answer. We can run a simple tasks, which gathers multiple coroutines:
import asyncio
async def test_1(dummy):
res1 = await foo1()
return res1
async def test_2(dummy):
res2 = await foo2()
return res2
async def multiple_tasks(dummy):
input_coroutines = [test_1(dummy), test_2(dummy)]
res = await asyncio.gather(*input_coroutines, return_exceptions=True)
return res
if __name__ == '__main__':
dummy = 0
res1, res2 = asyncio.get_event_loop().run_until_complete(multiple_tasks(dummy))
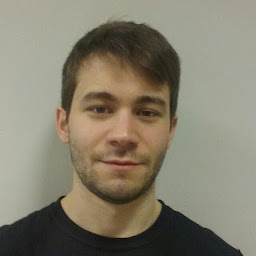
Author by
Federico Caccia
Software developer with passion in machine learning, blockchain and new technologies.
Updated on June 13, 2022Comments
-
Federico Caccia almost 2 years
I have the following code:
import asyncio async def test_1(): res1 = await foo1() return res1 async def test_2(): res2 = await foo2() return res2 if __name__ == '__main__': print(asyncio.get_event_loop().run_until_complete([test_1, test_2]))
But the last call to
.run_until_complete()
is not working. How can I perform an async call to multiple tasks using.run_until_complete()
?