MVC 5 How to use an object in rdlc report
Solution 1
You can use the ReportViewer object to render an RDLC to PDF or HTML. For my case (below) I wanted a PDF document and I returned it as a FileContentResult ActionResult. If you want it to return as a download use the File ActionResult (I've commented that out for your use).
public ActionResult GetPackingSlipPDF(int shipmentId)
{
var shipment = _inboundShipmentService.GetInboundShipmentById(shipmentId);
Warning[] warnings;
string mimeType;
string[] streamids;
string encoding;
string filenameExtension;
var viewer = new ReportViewer();
viewer.LocalReport.ReportPath = @"Labels\PackingSlip.rdlc";
var shipLabel = new ShippingLabel { ShipmentId = shipment.FBAShipmentId, Barcode = GetBarcode(shipment.FBAShipmentId) };
viewer.LocalReport.DataSources.Add(new ReportDataSource("ShippingLabel", new List<ShippingLabel> { shipLabel }));
viewer.LocalReport.Refresh();
var bytes = viewer.LocalReport.Render("PDF", null, out mimeType, out encoding, out filenameExtension, out streamids, out warnings);
return new FileContentResult(bytes, mimeType);
//return File(bytes, mimeType, shipment.FBAShipmentId + "_PackingSlip.pdf");
}
Rendering an RDLC report in HTML in ASP.NET MVC
Solution 2
You need to use ReportViewer. Here is a walkthrough for generating report from any Table or Stored Procedure. But from Visual Studio 2017 there is no ReportViewer tool by default. So to generate a report, first you need to configure several things.
Report Designer:
Go to Tools > Extensions and Updates. Then download and install Microsoft Rdlc Report Designer for Visual Studio.ReportViewer Controll:
Open package manager console and run this: install-package Microsoft.ReportingServices.ReportViewerControl.WebForms.- Add the ReportViewer Controll to ToolBox:
From ToolBox right click on General and select "Choose Items". After the loading is finished, click to Browse, then navigate to the project folder (the report viewer dll is located in the packages folder of the project). Go to Microsoft.ReportingServices.ReportViewerControl.WebForms\lib\net40 folder and add WebForms.dll. - From pm console run: install-package ReportViewerForMvc
-
A default "ReportViewerWebForm.aspx" will be added to the root location. Make sure it contains a ScriptManager and a ReportViewer. If not then simply add them from ToolBox or copy paste this:
<asp:ScriptManager ID="ScriptManager1" runat="server"> <Scripts> <asp:ScriptReference Assembly="ReportViewerForMvc" Name="ReportViewerForMvc.Scripts.PostMessage.js" /> </Scripts> </asp:ScriptManager> <rsweb:ReportViewer ID="ReportViewer1" runat="server"></rsweb:ReportViewer>
Now you need to create a DataSet to provide it to the report as its DataSource. So add a new DataSet (.xsd file). You can use both Tables or Stored Procedures in that Data Set. Simply open the Server Explorer and then drag and drop the data source (Table or Stored Procedure).
- Design a Report:
Add a new .rdlc file then go to the View > Report Data panel and add a new Data Source (choose that .xsd file), then choose which source from that .xsd file will be used as the DataSet for that report. -
Generate Report:
Now call the default "ReportViewerWebForm.aspx" like this:
From caller Controller:var reportViewer = new ReportViewer(); reportViewer.LocalReport.ReportPath = Server.MapPath("~/Reports/Reception/PatientMoneyReceipt.rdlc"); reportViewer.LocalReport.DataSources.Clear(); reportViewer.LocalReport.DataSources.Add(new ReportDataSource("ReportDataSet", _createEntryService.GetMoneyReceiptReport(model.Patient.PatientId))); reportViewer.LocalReport.Refresh(); reportViewer.ProcessingMode = ProcessingMode.Local; reportViewer.AsyncRendering = false; reportViewer.SizeToReportContent = true; reportViewer.ZoomMode = ZoomMode.FullPage; ViewBag.ReportViewer = reportViewer; return View();
From caller View (cshtml file):
@using ReportViewerForMvc ... @Html.ReportViewer(ViewBag.ReportViewer as Microsoft.Reporting.WebForms.ReportViewer)
In this line
reportViewer.LocalReport.DataSources.Add(new ReportDataSource("ReportDataSet", _createEntryService.GetMoneyReceiptReport(model.Patient.PatientId)));
the
ReportDataSet
is the data set name used when configuring that .rdlc file, and the_createEntryService.GetMoneyReceiptReport(model.Patient.PatientId))
is a service function that calls a stored procedure and return it as a DataTable.
Hope that helps.
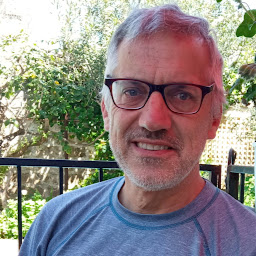
francisco Mc Manus
Updated on July 14, 2022Comments
-
francisco Mc Manus almost 2 years
this is my first question here.
I'm using VS Community 2015 and a MVC 5 project with Entity Framework 6. I use code first migration for data modeling.
I allready have reports using a view for each one. Each view uses a C# model for show report as a HTML5 web page.
Now I have to send the output to pdf, word, excel... For that I'll use RDLC but I don't know how set the object model as a dataset. The idea is send the same object that allready use the view for build the report. The data for the reports isn't.
Any idea or suggestion or tutorial how can I do that?
I'm very new to RDLC and I'd never use dataset before.
thanks
-
francisco Mc Manus over 7 yearsThanks Muhammad!, there is a lot new things for me here to study.
-
francisco Mc Manus over 7 yearsHow do I design the rdlc report? it ask for a dataset or data source... it forces me for use a data connection to SQL but I want a C# Model Object for data source.