MyBatis: Map String to boolean
10,085
Solution 1
What you need is a typeHandler for you Y/N Boolean type: (more explained here) Actual Handler:
public class YesNoBooleanTypeHandler extends BaseTypeHandler<Boolean> {
@Override
public void setNonNullParameter(PreparedStatement ps, int i, Boolean parameter, JdbcType jdbcType)
throws SQLException {
ps.setString(i, convert(parameter));
}
@Override
public Boolean getNullableResult(ResultSet rs, String columnName)
throws SQLException {
return convert(rs.getString(columnName));
}
@Override
public Boolean getNullableResult(ResultSet rs, int columnIndex)
throws SQLException {
return convert(rs.getString(columnIndex));
}
@Override
public Boolean getNullableResult(CallableStatement cs, int columnIndex)
throws SQLException {
return convert(cs.getString(columnIndex));
}
private String convert(Boolean b) {
return b ? "Y" : "N";
}
private Boolean convert(String s) {
return s.equals("Y");
}
}
Your usage:
<result property="myFlag" column="MY_FLAG" javaType="java.lang.Boolean" jdbcType="VARCHAR" typeHandler="com.foo.bar.YesNoBooleanTypeHandler" />
Solution 2
One way to do it would be to look at implementing a custom TypeHandler. http://www.mybatis.org/mybatis-3/configuration.html#typeHandlers.
Related videos on Youtube
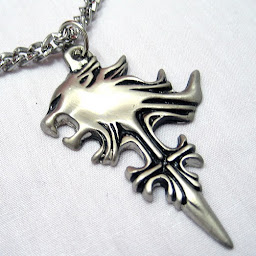
Author by
Julián Martínez
Updated on September 15, 2022Comments
-
Julián Martínez over 1 year
I have booleans inserted in my database as Y/N. When I try to map the result to a boolean java type, it always set it to a false in any case in my pojo.
Is there any way to map String to boolean? Here's my code:
<resultMap id="getFlag" type="MyPojo"> <result property="myFlag" column="MY_FLAG"/> </resultMap>
-
Pierre over 5 yearsRememember to register your Y/N TypeHandler upon creating your SqlSessionFactoryBean like this: factory.setTypeAliases(new Class[]{YesNoBooleanTypeHandler.class}). You must already configure your SqlSesionFactory in your applicationContext.xml [if using Spring XML] or Spring Config @Configuration [if using java annotations].