MyBatis! Passing multiple parameter to Mapper DAO
12,461
Solution 1
If you are using DAO implementation then you can do this by using a HashMap
. Just add the key-value pair in HashMap
and add it in function call and you can access it in the mapper.xml
using "key".
Solution 2
Use a Mapper.
interface Mapper
{
@Select( " select names from names_table where a = #{fieldA} and b = #{fieldB}" )
List<String> getNames( @Param("fieldA") String fieldA, @Param("fieldB") String fieldB)
}
The @Param tag allows you to specify what you can use to access the parameter in the sql map. This example shows a @Select tag, but it works the same as xml.
then change your code,
public List<String> getAllName() throws PersistenceException {
SqlSession session = sf.openSession();
try
{
Mapper mapper = session.getMapper(Mapper.class);
return mapper.getNames("a","b");
} finally {
session.close();
}
}
Read the user guide for more information.
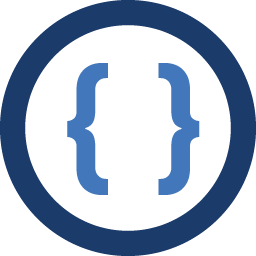
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am trying to send multiple parameter to Mapper function defined in DAO implementation but unable to send more than 1 parameter in the case when parameter are not holder of any class. I mean how can I modify the following code-
obj.getName(int a, int b);
In DAO implementation
public void getAllName() throws PersistenceException { SqlSession session = sf.openSession(); try { session.selectList("getNames"); } finally { session.close(); } }
I want to send a and b to query getNames.
Thanks in advance.