Node.js server "404 not found" message to 404.html page
19,966
Solution 1
H i ,
within your 404 case
response.writeHead(404, {"Content-Type": "text/plain"});
response.write("404 Not Found\n");
response.end();
You can change to
response.writeHead(404, {"Content-Type": "text/html"});
response.write(HTMLDATA);
response.end();
'HTMLDATA' being either a string of HTML or a reference to a file you have gathered.
response.writeHead()
is always set before the response.write()
.
Also see we have set the response type to 'text/html'
http://nodejs.org/api/http.html#http_class_http_serverresponse
Solution 2
response.writeHead(404, {
'Location': 'your/404/path.html'
//add other headers here...
});
response.end();
or with a single line
response.redirect('your/404/path.html');
Related videos on Youtube
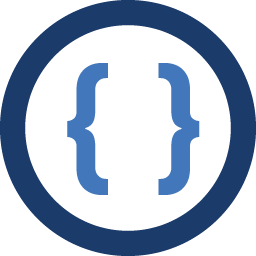
Author by
Admin
Updated on August 22, 2022Comments
-
Admin over 1 year
Im working with node.js and I would like to know how to display a 404.html instead of a "404 Not Found" message.
This is my server.js:
var http = require("http"), url = require("url"), path = require("path"), fs = require("fs") port = process.argv[2] || 8888; http.createServer(function(request, response) { var uri = url.parse(request.url).pathname , filename = path.join(process.cwd(), uri); path.exists(filename, function(exists) { if(!exists) { response.writeHead(404, {"Content-Type": "text/plain"}); response.write("404 Not Found\n"); response.end(); return; } if (fs.statSync(filename).isDirectory()) filename += 'public/Index/index.html'; fs.readFile(filename, "binary", function(err, file) { if(err) { response.writeHead(500, {"Content-Type": "text/plain"}); response.write(err + "\n"); response.end(); return; } response.writeHead(200); response.write(file, "binary"); response.end(); }); }); }).listen(parseInt(port, 10)); console.log("Static file server running at\n => http://localhost:" + port + "/\nCTRL + C to shutdown");
as you can see its just a static file server and I'm not using express.js or anything.
-
damphat over 10 yearsThis is the right one! Non redirect, the status is 404, content-type is text/html