Null object in c++
Solution 1
That's because ship
is not a pointer to Ship
, i.e. a Ship*
but it is a Ship
object itself; then you cannot convert it to 0
which is the null address for ... a pointer.
If you want a pointer to Ship
you should do
Ship* ship = new Ship;
// Catch a std::bad_alloc exception if new fails
Then if you obtain the pointer as a function argument you can test if it is null or not:
void foo(Ship* ship_pointer)
{
if(ship_pointer == 0)
// Oops pointer is null...
else
// Guess it's OK and use it.
}
Solution 2
From the look of the code you have decalred ship
as a member variable inside the class Crane
. If it is so, it can not be NULL. When Crane
object is created the ship
object is also constructed. You can test for NULL
only for pointers like that. I suggest you to read a book explaining the basic C++ syntax before proceeding further. If your intention is to check for whether ship is empty, you can provide a method called empty
in ship
class which returns a bool
and use that in the if
condition.
Solution 3
how to do this right?
Easy: Don't treat C++ like Java or some other language that forces an indirection onto you (reference<->object). C++ doesn't work like that. If you want an indirection you have to use something like pointers. There is really no difference between a class type and an int or double with recpect to copying, assignment, etc. There is no implicit indirection with class types.
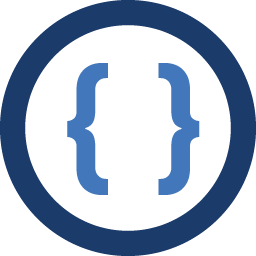
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
i am trying to check whether the ship object is null, but i got an error message
Crane.cpp:18: error: could not convert ‘((Crane*)this)->Crane::ship.Ship::operator=(((const Ship&)(& Ship(0, std::basic_string, std::allocator >(((const char*)"arrive"), ((const std::allocator&)((const std::allocator)(& std::allocator())))), std::basic_string, std::allocator >(((const char)"Ship"), ((const std::allocator&)((const std::allocator*)(& std::allocator()))))))))’ to ‘bool’
Crane::Crane(int craneId, int craneStatus, bool free, Ship ship) { setCraneId(craneId); setCraneStatus(craneStatus); setFree(free); setShip(ship); } Crane::Crane(){} Crane::~Crane(){} void Crane::print() { cout << "Crane Id: " << craneId << endl; cout << "Crane Status: " << craneStatus << endl; cout << "Crane is free: " << free << endl; if (ship = NULL) //this is the problem { cout << " " << endl; } else { ship.print();//i have another print method in the Ship class } }
i have tried
if (ship == NULL)
but i get this error message
Crane.cpp:18: error: no match for ‘operator==’ in ‘((Crane*)this)->Crane::ship == 0’
how to do this right?