Obtaining user roles in servlet application using keycloak
10,987
Solution 1
@Shiva's answer did not work for me. getRealmAccess() was returning null. we had to use the following:
KeycloakPrincipal principal = (KeycloakPrincipal) request.getUserPrincipal();
String clientId = "securesite";
principal.getKeycloakSecurityContext().getToken().getResourceAccess(clientId).getRoles();
Solution 2
If the servlet is protected by keyclaok then you can use the following API to get the KeycloakSecurityContext
and then access the Set
of roles to modify it.
KeycloakPrincipal principal = (KeycloakPrincipal) request.getUserPrincipal();
principal.getKeycloakSecurityContext().getToken().getRealmAccess().getRoles().add("Test-Role");
A sample servlet request might look like this.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
@SuppressWarnings("rawtypes")
KeycloakPrincipal principal = (KeycloakPrincipal)request.getUserPrincipal();
if (principal != null) {
//user has a valid session, we can assign role on the fly like this
principal.getKeycloakSecurityContext().getToken().getRealmAccess().getRoles().add("Test-Role");
}
}
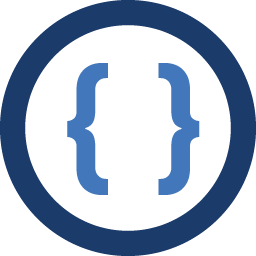
Author by
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I'm using keycloak to protect my servlet. I have to add new roles and assign them to users dynamically. It works in keycloak using admin API, but I can't figure out how to obtain the roles for specific user in a servlet.
I tried this solution, but I get empty set:
protected void doPost(HttpServletRequest request, HttpServletResponse response) { ... KeycloakSecurityContext context = (KeycloakSecurityContext)request.getAttribute(KeycloakSecurityContext.class.getName()); Set<String> roles = AdapterUtils.getRolesFromSecurityContext((RefreshableKeycloakSecurityContext) context); ... }