OKhttp PUT example
Solution 1
Change your .post
with .put
public void putRequestWithHeaderAndBody(String url, String header, String jsonBody) {
MediaType JSON = MediaType.parse("application/json; charset=utf-8");
RequestBody body = RequestBody.create(JSON, jsonBody);
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(url)
.put(body) //PUT
.addHeader("Authorization", header)
.build();
makeCall(client, request);
}
Solution 2
OkHttp Version 2.x
If you're using OkHttp Version 2.x, use the following:
OkHttpClient client = new OkHttpClient();
RequestBody formBody = new FormEncodingBuilder()
.add("Key", "Value")
.build();
Request request = new Request.Builder()
.url("http://www.foo.bar/index.php")
.put(formBody) // Use PUT on this line.
.build();
Response response = client.newCall(request).execute();
if (!response.isSuccessful()) {
throw new IOException("Unexpected response code: " + response);
}
System.out.println(response.body().string());
OkHttp Version 3.x
As OkHttp version 3 replaced FormEncodingBuilder
with FormBody
and FormBody.Builder()
, for versions 3.x you have to do the following:
OkHttpClient client = new OkHttpClient();
RequestBody formBody = new FormBody.Builder()
.add("message", "Your message")
.build();
Request request = new Request.Builder()
.url("http://www.foo.bar/index.php")
.put(formBody) // PUT here.
.build();
try {
Response response = client.newCall(request).execute();
// Do something with the response.
} catch (IOException e) {
e.printStackTrace();
}
Solution 3
Use put
method instead of post
Request request = new Request.Builder()
.url(url)
.put(body) // here we use put
.addHeader("Authorization", header)
.build();
Related videos on Youtube
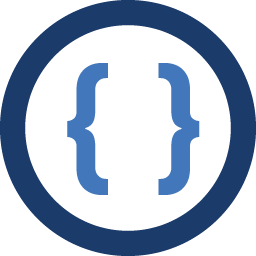
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
My requirement is to use
PUT
, send a header and a body to server which will update something in the database.I just read okHttp documentation and I was trying to use their
POST
example but it doesn't work for my use case (I think it might be because the server requires me to usePUT
instead ofPOST
).This is my method with
POST
:public void postRequestWithHeaderAndBody(String url, String header, String jsonBody) { MediaType JSON = MediaType.parse("application/json; charset=utf-8"); RequestBody body = RequestBody.create(JSON, jsonBody); OkHttpClient client = new OkHttpClient(); Request request = new Request.Builder() .url(url) .post(body) .addHeader("Authorization", header) .build(); makeCall(client, request); }
I have tried to search for okHttp example using
PUT
with no success, if I need to usePUT
method is there anyway to use okHttp?I'm using okhttp:2.4.0 (just in case), thanks on any help!
-
B.shruti over 5 yearsWhat if the request doesn't have a body , I mean how to send a put request using OKHttp without a request body ?